Transforming Images into Markdown: A Guide to LlamaOCR
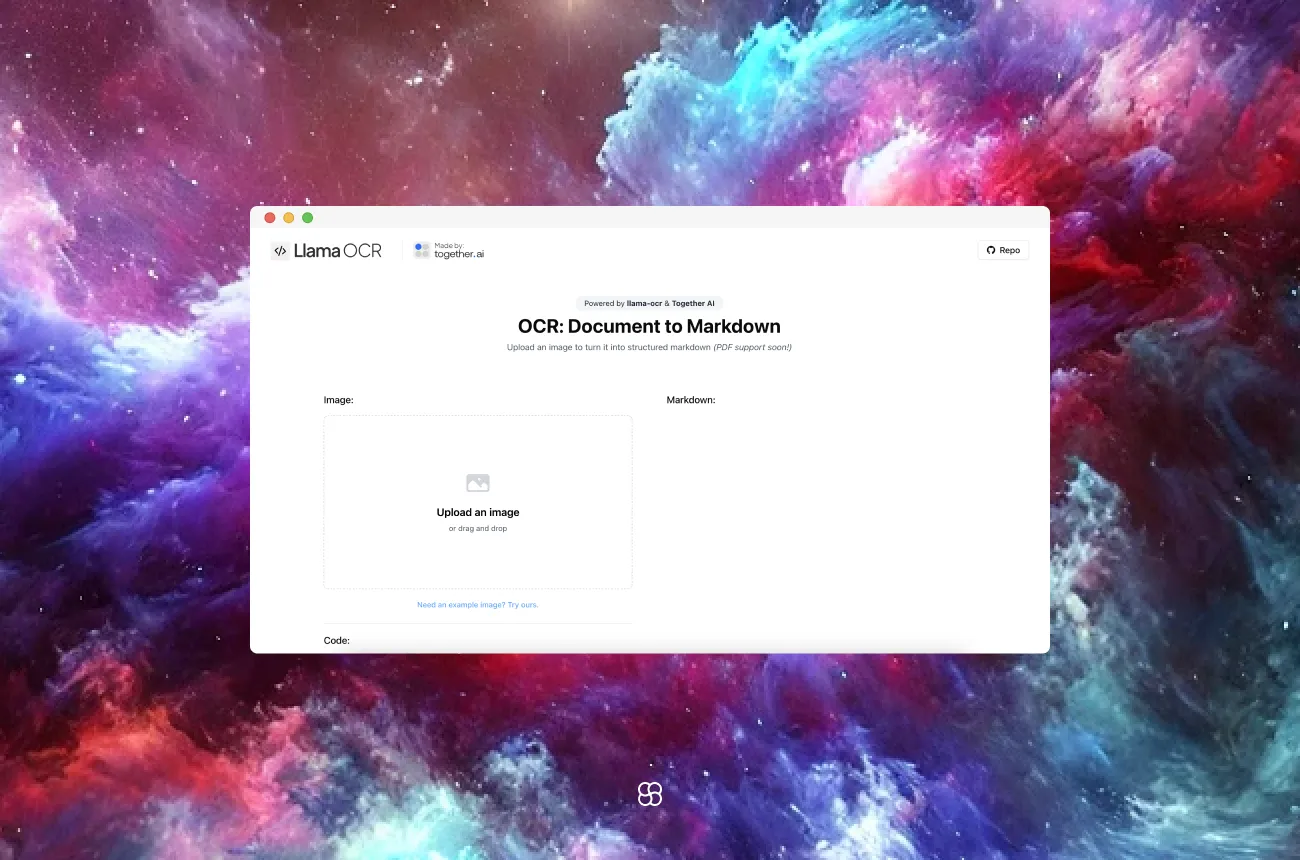
In an era defined by digital transformation, the ability to convert physical documents into editable, structured formats is not just a convenience—it’s a necessity. LlamaOCR, an open-source Optical Character Recognition (OCR) tool powered by the Llama 3.2 Vision model, offers a powerful and innovative solution. By converting images directly into Markdown text, LlamaOCR simplifies workflows, maintains formatting, and accelerates productivity. You can also test it here.
This comprehensive guide walks you through LlamaOCR’s features, benefits, and advanced applications, complete with step-by-step instructions and ready-to-use code snippets to supercharge your projects.
What Is LlamaOCR?
LlamaOCR excels at extracting text from images, even when dealing with complex layouts like tables, receipts, and multi-format documents. Its standout feature is direct Markdown conversion, which preserves formatting and structure, ensuring that your digital output mirrors the original. Whether you're a developer or a tech enthusiast, LlamaOCR is a game-changer.
Key Benefits
Here’s why LlamaOCR stands out among OCR solutions:
- High Accuracy: Powered by advanced AI, LlamaOCR extracts text with remarkable precision, even from cluttered or unconventional layouts.
- Markdown-First Design: Outputs directly in Markdown format, saving you time by preserving the structure and making integration effortless.
- Easy Integration: Packaged as an npm module, it integrates seamlessly with JavaScript or TypeScript projects, ensuring minimal friction during setup.
Getting Started
Step 1: Installation
Begin by installing the npm package. Make sure Node.js is installed on your system, then run:
npm install llama-ocr
Step 2: Obtain an API Key
LlamaOCR uses the Llama 3.2 Vision model endpoint provided by Together AI. To access this feature, register on their platform to get a free API key.
Initial Setup
Step 1: Import the Library
Add the ocr
function to your project to begin processing images:
import { ocr } from 'llama-ocr';
Step 2: Configure Environment Variables
For security, store your API key in an environment variable:
export TOGETHER_API_KEY=your_api_key_here
With the library imported and your API key secured, you’re ready to perform OCR.
Performing OCR on an Image
Extracting text from an image is simple with LlamaOCR. Here’s how:
const markdown = await ocr({
filePath: './path_to_your_image.jpg', // Specify the image path
apiKey: process.env.TOGETHER_API_KEY, // Securely pass your API key
});
console.log(markdown);
This function reads the image file, processes it through the Llama 3.2 Vision model, and returns the text as Markdown, complete with the original document’s structure.
Automating OCR with an Agent
Let’s take it a step further and automate OCR processing for repetitive tasks.
Step 1: Initialize a Node.js Project
Set up a new project and install dependencies:
npm init -y
npm install llama-ocr
Step 2: Build the OCR Script
Create a script (ocrAgent.js
) to process images and save the extracted text as a Markdown file:
import { ocr } from 'llama-ocr';
import fs from 'fs';
const apiKey = process.env.TOGETHER_API_KEY;
const imagePath = './path_to_your_image.jpg';
async function runOCR() {
try {
const markdown = await ocr({ filePath: imagePath, apiKey });
fs.writeFileSync('output.md', markdown);
console.log('OCR completed successfully. Output saved to output.md');
} catch (error) {
console.error('Error during OCR process:', error);
}
}
runOCR();
Step 3: Execute the Script
Run the script using Node.js:
node ocrAgent.js
This processes the specified image and saves the extracted Markdown to output.md
.
Advanced Applications
LlamaOCR’s potential extends far beyond single-image processing. Here are some advanced use cases:
1. Batch Processing
Automate OCR for multiple files by iterating over a directory of images:
import fs from 'fs';
import path from 'path';
import { ocr } from 'llama-ocr';
const directoryPath = './images';
const apiKey = process.env.TOGETHER_API_KEY;
async function batchProcessOCR() {
const files = fs.readdirSync(directoryPath);
for (const file of files) {
const filePath = path.join(directoryPath, file);
try {
const markdown = await ocr({ filePath, apiKey });
const outputFilePath = `./output/${path.basename(file, path.extname(file))}.md`;
fs.writeFileSync(outputFilePath, markdown);
console.log(`Processed ${file} successfully.`);
} catch (error) {
console.error(`Error processing ${file}:`, error);
}
}
}
batchProcessOCR();
2. Web Application Integration
Incorporate LlamaOCR into your web app, allowing users to upload images and receive instant Markdown conversion.
3. Expand Document Formats
While LlamaOCR currently supports images, upcoming updates aim to include PDF compatibility, broadening its utility for digitizing scanned documents.
Why Markdown?
Markdown is a developer-friendly format that combines simplicity and flexibility. By outputting directly in Markdown, LlamaOCR eliminates manual formatting, saving time and effort while enabling seamless integration into documentation workflows, blogs, or apps.
Final Thoughts
LlamaOCR is more than an OCR tool—it’s a productivity powerhouse. Whether you’re digitizing archives, automating workflows, or building OCR-enabled apps, LlamaOCR delivers high accuracy, effortless integration, and the unmatched convenience of Markdown output.
By adopting LlamaOCR, you can take your document digitization to the next level, saving time, enhancing accuracy, and focusing on what truly matters: creating value for your projects.
Cohorte Team
January 7, 2025