Tools of the Trade: Mastering Tool Integration in SmolAgents (Part 2)
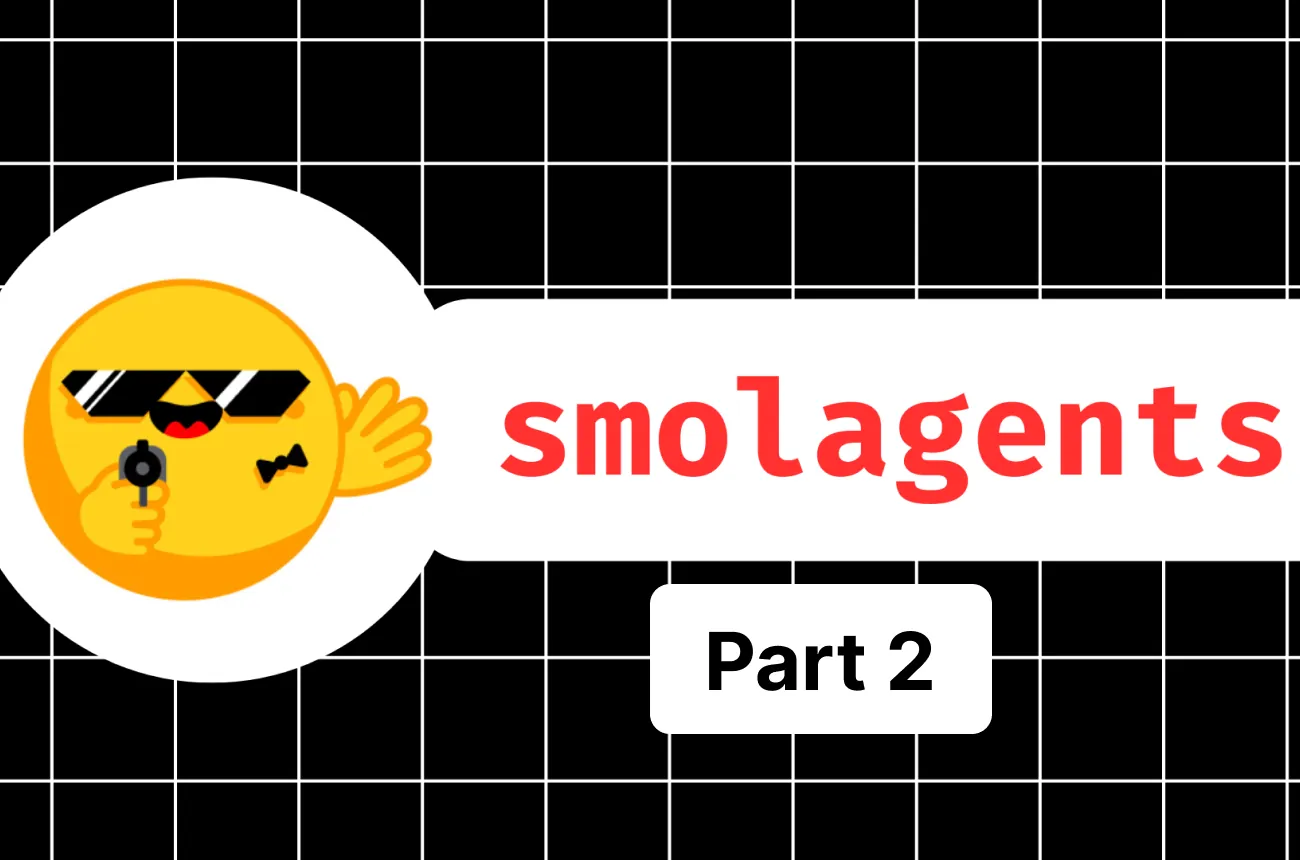
Introduction: The Power of Tools in Agentic Systems
Imagine a carpenter with no tools—only a good sense of woodcraft. Their potential is severely limited. The same applies to AI agents. Without tools, even the smartest agent struggles to perform meaningful tasks in the real world.
In SmolAgents, tools are the agent's way of interacting with the world: they fetch data, perform calculations, and execute commands. From web searches to database queries, tools empower agents to go beyond text generation and truly act.
This guide dives deep into tools: what they are, how to build them, and how to use them effectively. By the end, you'll master the art of tool integration in SmolAgents.
1. What Are Tools in SmolAgents?
In SmolAgents, tools are functions with metadata that describe:
- What the tool does (description).
- What inputs it requires (input schema).
- What outputs it provides (output schema).
These details help the agent understand when and how to use the tool. Tools act as the bridge between the agent’s reasoning and the actions it performs.
2. Default Toolbox: Out-of-the-Box Utility
SmolAgents come with a set of pre-built tools, ready to plug and play. Here’s a glimpse of what’s included:
- DuckDuckGoSearchTool: Performs web searches.
- PythonInterpreterTool: Executes Python code securely.
- Transcriber: Converts audio to text using Whisper-Turbo.
To add these to your agent:
from smolagents import CodeAgent, HfApiModel
model = HfApiModel("meta-llama/Llama-3.3-70B-Instruct")
agent = CodeAgent(tools=[], model=model, add_base_tools=True)
result = agent.run("Search for the latest AI news.")
print(result)
3. Creating Custom Tools
Default tools are great, but most real-world applications demand custom tools tailored to specific tasks. Let’s explore how to create and use your own tools.
Step 1: Define a Basic Tool
Here’s a tool that retrieves the most downloaded model for a given task on Hugging Face Hub:
rom huggingface_hub import list_models
from smolagents import tool
@tool
def model_download_tool(task: str) -> str:
"""
Returns the most downloaded model for a specific task on the Hugging Face Hub.
Args:
task: The task (e.g., "text-classification").
"""
most_downloaded_model = next(iter(list_models(filter=task, sort="downloads", direction=-1)))
return most_downloaded_model.id
Step 2: Use the Tool in an Agent
Add the tool to an agent and see it in action:
agent = CodeAgent(tools=[model_download_tool], model=model)
result = agent.run("Find the most downloaded model for text-to-video tasks.")
print(result)
Output Example
The most downloaded model for text-to-video tasks is ByteDance/AnimateDiff-Lightning.
4. Advanced Tool Features
A. Input and Output Validation
To ensure reliable execution, tools in SmolAgents can enforce strict input and output validation using type hints and docstrings:
@tool
def add_numbers(a: int, b: int) -> int:
"""
Adds two numbers together.
Args:
a: The first number.
b: The second number.
"""
return a + b
If the agent tries to call add_numbers
with invalid inputs, it will trigger an error.
B. Logging and Error Handling
To help the agent debug itself, tools can log useful information:
@tool
def divide_numbers(a: float, b: float) -> float:
"""
Divides two numbers.
Args:
a: Numerator.
b: Denominator.
"""
if b == 0:
raise ValueError("Division by zero is not allowed.")
return a / b
The agent can learn from error messages and retry with corrected inputs.
C. Complex Tools: Using External APIs
Let’s build a tool that retrieves weather data:
import requests
from smolagents import tool
@tool
def get_weather(city: str) -> str:
"""
Retrieves the current weather for a city.
Args:
city: Name of the city.
"""
api_key = "<YOUR_API_KEY>"
url = f"http://api.openweathermap.org/data/2.5/weather?q={city}&appid={api_key}"
response = requests.get(url)
data = response.json()
return f"The weather in {city} is {data['weather'][0]['description']} with a temperature of {data['main']['temp']}°K."
5. Sharing Tools
SmolAgents supports sharing tools on Hugging Face Hub, making them reusable for others.
Step 1: Push Your Tool to the Hub
model_download_tool.push_to_hub("<username>/model-download-tool", token="<YOUR_HF_TOKEN>")
Step 2: Load Tools from the Hub
Anyone can load your tool using:
from smolagents import load_tool
tool = load_tool("<username>/model-download-tool", trust_remote_code=True)
6. Multi-Tool Agents
Agents can use multiple tools simultaneously, enabling complex workflows.
Scenario: An Agentic Research Assistant
Let’s combine:
- A web search tool.
- A webpage summarization tool.
- A weather tool.
Here’s how:
from smolagents import CodeAgent, DuckDuckGoSearchTool
@tool
def summarize_webpage(url: str) -> str:
"""
Summarizes a webpage given its URL.
"""
response = requests.get(url)
content = response.text
return content[:200] # Simplified example: return the first 200 characters
agent = CodeAgent(
tools=[DuckDuckGoSearchTool(), summarize_webpage, get_weather],
model=model,
add_base_tools=True
)
result = agent.run("Search for the latest AI trends, summarize the top result, and get the weather in New York.")
print(result)
7. Best Practices for Tools
- Clarity is Key: Clearly describe tool capabilities in the
description
field. - Minimal Toolset: Avoid overwhelming the agent with too many tools.
- Error Messages: Provide actionable error messages to guide the agent.
- Security: Validate inputs and restrict imports for safety.
- Test Extensively: Simulate edge cases to ensure tools behave as expected.
8. Debugging Tool Usage
Sometimes, agents misuse tools due to unclear descriptions or unexpected inputs. Here’s how to fix that:
- Inspect Logs: Use
agent.logs
to identify errors. - Improve Descriptions: Add specific examples to tool docstrings.
- Switch Models: Use more capable models for complex reasoning.
9. Conclusion: Empowering Agents with Tools
Tools are the building blocks of SmolAgents. They turn static AI into dynamic problem solvers capable of interacting with the world. By mastering tool integration, you unlock the full potential of agentic systems.
Whether you’re creating simple tools for personal projects or complex, multi-tool workflows for enterprise applications, SmolAgents gives you the flexibility and power to build with confidence.
Start small, think big, and let your agents take the wheel. The future of AI is interactive, and you’re now equipped to lead the charge. Check out this source for more information about smolagents.
Cohorte Team
February 25, 2025