PromptLayer 101: The Beginner’s Guide to Supercharging Your LLM Workflow
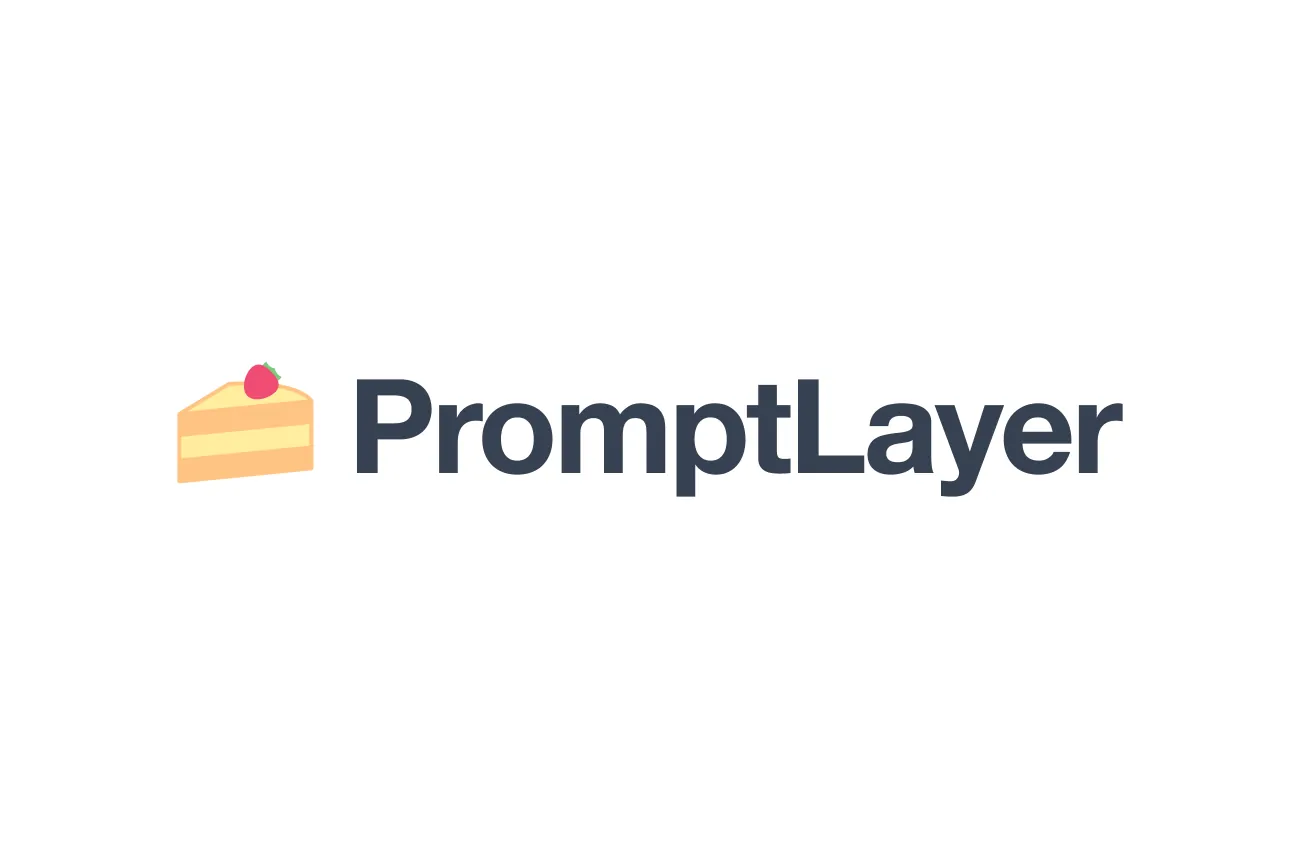
Introduction: Why PromptLayer?
Prompt engineering is the art and science of crafting the right instructions (or prompts) to guide Large Language Models (LLMs) like GPT into giving you the best possible results. But once your prompts get complicated, managing, testing, and iterating on them becomes overwhelming. That’s where PromptLayer shines.
Think of PromptLayer as your prompt control center—a platform that tracks, tests, and optimizes your LLM prompts. It’s the missing piece that bridges creativity with engineering, allowing developers and prompt engineers to work smarter, not harder.
In this guide, we’ll introduce the fundamental features of PromptLayer and teach you how to level up your LLM projects.
Core Concepts: What Does PromptLayer Do?
- Prompt Management:
Store, organize, and version-control prompts. Forget hardcoding prompts into your applications—PromptLayer lets you keep them in a central "Prompt Registry," where non-technical teammates can collaborate too.
- Prompt Iteration:
Experiment with different prompt versions without redeploying code. Tweak prompts directly in the dashboard and instantly test their performance.
- Analytics and Logging:
Track every request and response made to your LLMs. Get insights into performance, usage patterns, and cost.
- A/B Testing:
Safely test new prompts in production by splitting user traffic between different prompt versions.
- Evaluations:
Run batch tests to backtest prompts, catch regressions, and validate updates using real or synthetic datasets.
Step 1: Setting Up PromptLayer
1. Create an Account
Sign up at promptlayer.com. After creating your account, you’ll receive an API key from the settings page.
2. Install the SDK
PromptLayer integrates seamlessly with Python and JavaScript. For Python:
pip install promptlayer
pip install openai
3. Wrap Your OpenAI SDK
PromptLayer acts as a wrapper for OpenAI’s SDK, logging requests and responses automatically. Update your code like this:
from promptlayer import PromptLayer
# Initialize PromptLayer client
promptlayer_client = PromptLayer(api_key="your_promptlayer_api_key")
# Wrap OpenAI
OpenAI = promptlayer_client.openai.OpenAI
client = OpenAI()
response = client.chat.completions.create(
model="gpt-3.5-turbo",
messages=[
{"role": "system", "content": "You are a helpful assistant."},
{"role": "user", "content": "How does PromptLayer work?"}
]
)
print(response["choices"][0]["message"]["content"])
Step 2: Managing Prompts with the Prompt Registry
What’s the Prompt Registry?
The Prompt Registry is a CMS (Content Management System) for prompts. It decouples prompts from your code, so you can edit them in one place without touching your application.
Here’s how you can create your first prompt template:
- Go to the Prompt Registry on the dashboard.
- Click Create Template and name it (e.g., "ai-poet").
- Add the system prompt:
You are a skilled poet specializing in haiku. Write a haiku based on {topic}.
- Save the template.
You can now retrieve and run this prompt programmatically:
input_variables = {"topic": "winter mornings"}
response = promptlayer_client.run(
prompt_name="ai-poet",
input_variables=input_variables
)
print(response["raw_response"]["choices"][0]["message"]["content"])
Step 3: Iterating and Testing Prompts
A/B Testing
Want to test two prompt variations? Assign release labels like "prod" and "dev" to your prompt versions:
response = promptlayer_client.run(
prompt_name="ai-poet",
input_variables={"topic": "autumn leaves"},
prompt_release_label="dev"
)
Split traffic between the two versions to gather real-world performance data.
Batch Testing
Evaluate prompts against large datasets to simulate real-world performance. Create a dataset from historical LLM requests or upload a JSON/CSV file:
[
{"topic": "spring blossoms"},
{"topic": "summer heat"}
]
Run batch evaluations directly in the dashboard or programmatically.
Step 4: Tracking, Logging, and Analytics
PromptLayer automatically logs every API request and response. Open the Logs page to see:
- Requests: What was sent to the LLM.
- Responses: What the LLM returned.
- Cost: How much each request cost.
You can filter logs by tags, metadata, or user IDs. Example:
promptlayer_client.track.metadata(
request_id=response["request_id"],
metadata={"user_id": "123", "environment": "production"}
)
Conclusion
With PromptLayer, managing prompts becomes as easy as managing code. From version control to A/B testing, logging, and analytics, it’s a one-stop shop for building and maintaining robust LLM applications.
Start small—track your first LLM request, create a template, and explore the analytics.
Cohorte Team
February 24, 2025