Mastering LangGraph: A Step-by-Step Guide to Building Intelligent AI Agents with Tool Integration
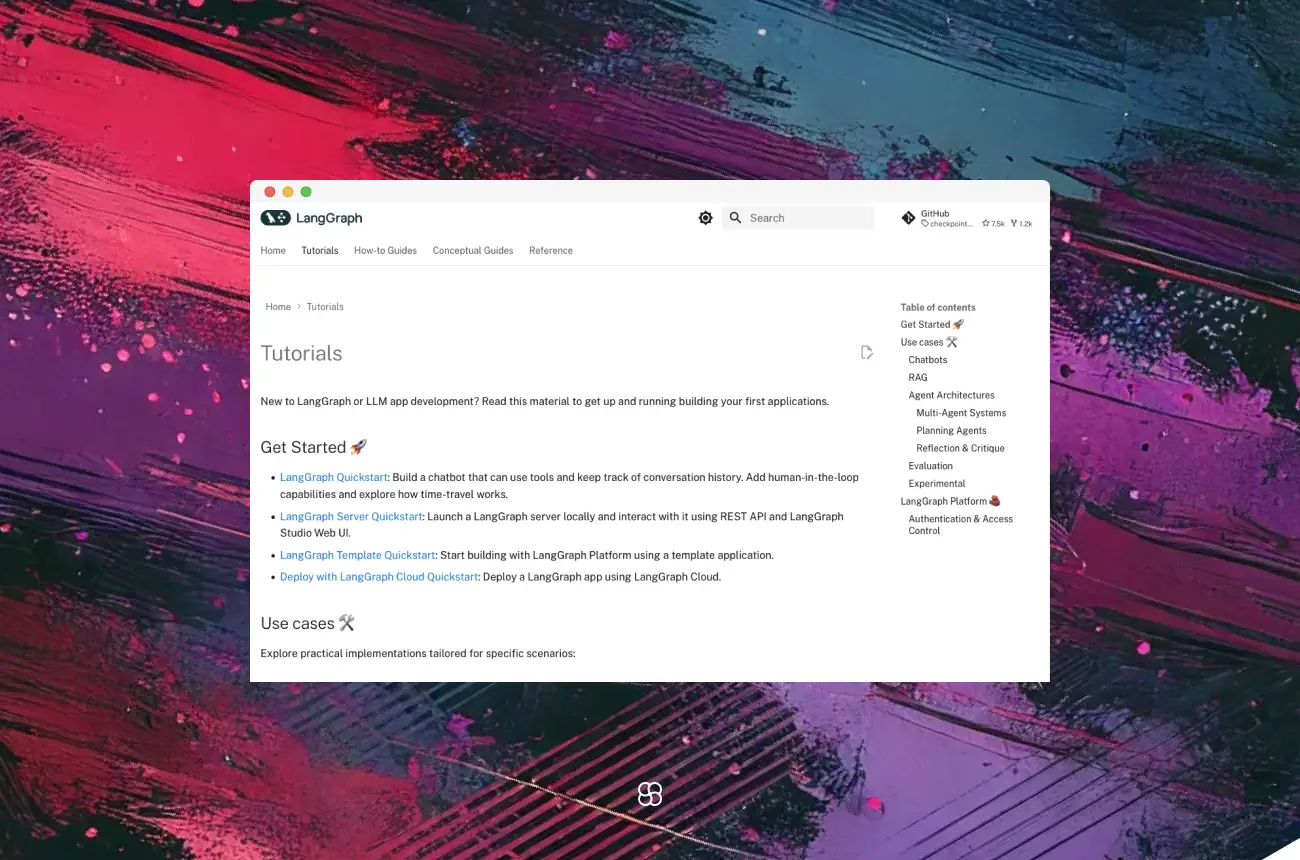
LangGraph is a versatile framework that enables developers to create sophisticated AI agents by combining natural language processing with graph-based reasoning. By representing workflows as graphs, LangGraph allows for complex decision-making processes and seamless integration of various tools, enhancing the agent’s capabilities beyond simple query responses.
Getting Started with LangGraph
Installation and Setup
To begin building with LangGraph, ensure that your development environment is prepared. LangGraph is compatible with Python 3.8 and above. You can install it using pip:
pip install -U langgraph
First Steps
After installation, initialize a LangGraph project by importing the necessary modules and setting up the basic structure:
from langgraph.graph import StateGraph, START, END
from langgraph.prebuilt import create_react_agent
from langchain_openai import ChatOpenAI
from langchain_core.tools import tool
from typing import TypedDict, Annotated
from langgraph.graph.message import add_messages
# Define the state structure
class State(TypedDict):
messages: Annotated[list, add_messages]
# Initialize the graph
graph_builder = StateGraph(State)
Step-by-Step Example: Building a Sophisticated Agent with Tool Integration
Defining the Agent and Its Functionality
In this example, we’ll build an AI agent capable of performing complex tasks by integrating external tools. The agent will utilize a knowledge graph to store information and will be able to answer user queries by retrieving relevant data from this graph and interacting with external tools as needed.
1. Define and Integrate Tools
Tools extend the agent’s capabilities by allowing it to interact with external systems or APIs. For instance, we can define a tool that fetches weather information:
@tool
def get_weather(location: str):
"""Fetches the current weather for a given location."""
# Placeholder implementation
if location.lower() == "san francisco":
return "It's 60 degrees and foggy."
else:
return "Weather data not available for the specified location."
We can then bind this tool to our language model:
llm = ChatOpenAI(model="gpt-4")
llm_with_tools = llm.bind_tools([get_weather])
2. Implement the Agent’s Workflow
Using LangGraph, we define the workflow of our agent by specifying the sequence of operations and how they interact with the state. We’ll create nodes for processing user input, deciding on tool usage, and generating responses:
def process_input(state: State):
user_message = state["messages"][-1]
# Process the user input as needed
return {"messages": state["messages"]}
def decide_action(state: State):
# Determine if a tool is needed based on the user input
if "weather" in state["messages"][-1].content.lower():
return {"action": "use_tool", "tool_name": "get_weather"}
else:
return {"action": "respond"}
def execute_tool(state: State):
tool_name = state.get("tool_name")
if tool_name == "get_weather":
location = extract_location(state["messages"][-1].content)
tool_response = get_weather(location)
state["messages"].append({"role": "tool", "content": tool_response})
return state
def generate_response(state: State):
# Generate a response using the language model
response = llm_with_tools.invoke(state["messages"])
state["messages"].append({"role": "assistant", "content": response})
return state
We then add these nodes to our graph and define the workflow:
graph_builder.add_node("process_input", process_input)
graph_builder.add_node("decide_action", decide_action)
graph_builder.add_node("execute_tool", execute_tool)
graph_builder.add_node("generate_response", generate_response)
graph_builder.add_edge(START, "process_input")
graph_builder.add_edge("process_input", "decide_action")
graph_builder.add_edge("decide_action", "execute_tool", condition=lambda state: state["action"] == "use_tool")
graph_builder.add_edge("decide_action", "generate_response", condition=lambda state: state["action"] == "respond")
graph_builder.add_edge("execute_tool", "generate_response")
graph_builder.add_edge("generate_response", END)
3. Run the Agent
With the graph defined, we compile and run the agent:
graph = graph_builder.compile()
user_input = "What's the weather in San Francisco?"
initial_state = {"messages": [{"role": "user", "content": user_input}]}
for event in graph.stream(initial_state):
for value in event.values():
print("Assistant:", value["messages"][-1]["content"])
This setup allows the agent to process user input, decide whether to use an external tool, execute the tool if necessary, and generate a response based on the combined information.
Examples: How the Community is Using LangGraph
LangGraph has found its place in diverse projects, showing its adaptability in building sophisticated AI systems. Here’s how developers are leveraging its capabilities:
Decision-Making Systems:
LangGraph is being used to create decision-making systems that replicate human-like reasoning. By defining workflows through nodes and edges, developers can build agents capable of managing complex scenarios, from customer support interactions to operational planning.
Code Generation and Analysis:
In the world of programming, LangGraph powers tools that generate, analyze, and optimize code. These agents use graph workflows to evaluate code structures, suggest improvements, or even create executable code snippets, streamlining the software development process.
AI Travel Agents:
AI-driven travel agents built on LangGraph are planning trips with precision. They pull real-time data on flights, hotels, and itineraries while incorporating user preferences. By integrating APIs and automating logistics, these agents are transforming travel planning into a seamless experience.
LangGraph’s community demonstrates its value as a foundational tool for creating AI systems that bridge reasoning, tools, and dynamic user interaction. These examples highlight its flexibility across industries and its ability to address complex problem-solving requirements.
Final Thoughts
Building a sophisticated LangGraph agent involves defining a structured workflow that integrates external tools and processes user inputs intelligently. By leveraging LangGraph’s capabilities, developers can create AI agents that perform complex tasks, interact with various data sources, and provide nuanced responses, significantly enhancing the user experience.
Cohorte Team
December 25, 2024