How to Build a Smart Web-Scraping AI Agent with LangGraph and Selenium
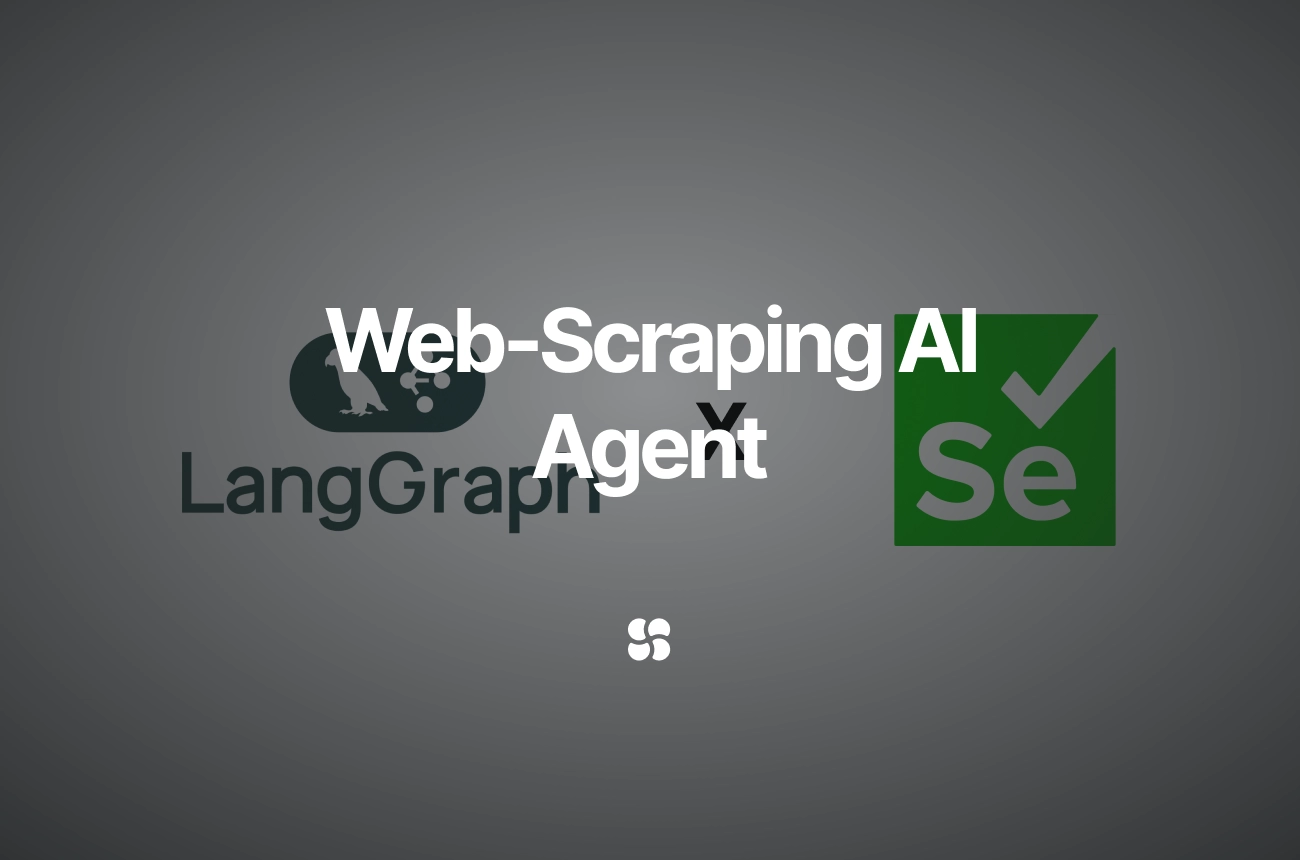
In today’s data-driven world, creating intelligent agents that can autonomously scrape, analyze, and collect information from the web is an invaluable skill. This guide will walk you through the process of building a simple AI agent using an agent framework like LangGraph combined with Selenium for web scraping. We'll cover the presentation of the framework, getting started with installation and setup, and then dive into a step-by-step example with code snippets. Finally, we'll wrap up with some final thoughts on how to expand and improve your agent.
Presentation of the Framework
LangGraph is an agent framework designed to streamline the creation and orchestration of AI agents. It allows you to define modular "nodes" or tasks that your agent can execute. Each node can encapsulate a specific function—like data extraction, processing, or even decision-making. By integrating tools like Selenium, LangGraph agents can not only process data internally but also interact with the web dynamically. Selenium acts as a powerful browser automation tool, enabling your agent to navigate web pages, simulate user interactions, and extract relevant information in a smart and automated way.
Getting Started
Installation and Setup
Before diving into code, ensure you have Python installed and then install the necessary packages. Open your terminal and run:
pip install langgraph selenium
Additionally, download the appropriate WebDriver (e.g., ChromeDriver) for your browser and ensure it's accessible in your system’s PATH or specify its location directly in your code.
First Steps: Setting Up Selenium
Selenium requires a WebDriver to interact with your browser. Here’s a snippet to initialize a Selenium WebDriver:
from selenium import webdriver
from selenium.webdriver.chrome.service import Service
def init_driver():
# Update the path to your chromedriver
service = Service(executable_path='/path/to/chromedriver')
driver = webdriver.Chrome(service=service)
return driver
This simple function sets up Selenium, making it ready for use in your agent.
Step-by-Step Example: Building a Simple Agent
Let’s build a basic web-scraping agent using LangGraph and Selenium. The goal is to navigate to a given URL, extract the page title, and then return that information.
Step 1: Import Libraries and Initialize the Framework
First, import the necessary libraries and define your agent class based on LangGraph’s structure:
from langgraph import Agent, Node
from selenium import webdriver
from selenium.webdriver.chrome.service import Service
from selenium.webdriver.common.by import By
import time
# Initialize Selenium WebDriver
def init_driver():
service = Service(executable_path='/path/to/chromedriver') # Set your path
driver = webdriver.Chrome(service=service)
return driver
Step 2: Define a Scraping Node
Create a node that will be responsible for scraping data. In this example, the node will extract the page title:
class ScrapingNode(Node):
def process(self, input_data):
url = input_data.get("url", "https://example.com")
driver = init_driver()
driver.get(url)
time.sleep(2) # Wait for the page to load completely
# Extract page title as a demonstration
title = driver.title
driver.quit()
return {"title": title}
Step 3: Build the AI Agent
Now, define your AI agent by incorporating the scraping node. This agent can then orchestrate various tasks, but for simplicity, it will just handle the scraping process:
class WebScraperAgent(Agent):
def __init__(self):
super().__init__()
self.add_node(ScrapingNode("scrape"))
def run_agent(self, url):
input_data = {"url": url}
result = self.process_node("scrape", input_data)
return result
if __name__ == "__main__":
agent = WebScraperAgent()
result = agent.run_agent("https://example.com")
print("Page title:", result.get("title"))
This code creates an instance of WebScraperAgent
that, when executed, uses Selenium to navigate to a specified URL, extract the page title, and print it out.
Final Thoughts
Building an AI agent using a framework like LangGraph in tandem with Selenium provides a robust foundation for more complex automation tasks. While this guide covers a simple use case, consider expanding your agent with additional nodes that can:
- Process extracted data with natural language processing tools.
- Integrate with databases for storing and analyzing information.
- Handle dynamic web pages with advanced Selenium techniques.
- Incorporate error handling and logging for more robust operation.
By leveraging modular frameworks, you can quickly iterate and expand your agent’s capabilities, making it a versatile tool in your data collection and analysis toolkit. Remember, ethical considerations are paramount when scraping web data—always respect a website's terms of service and robot exclusion protocols.
This guide should serve as a stepping stone into the world of AI-driven web automation, offering a blend of practical code examples and conceptual understanding to help you build your own intelligent agents. Happy coding!
Cohorte Team
March 28, 2025