Fine-Tuning and Evaluations: Mastering Prompt Iteration with PromptLayer (Part 2)
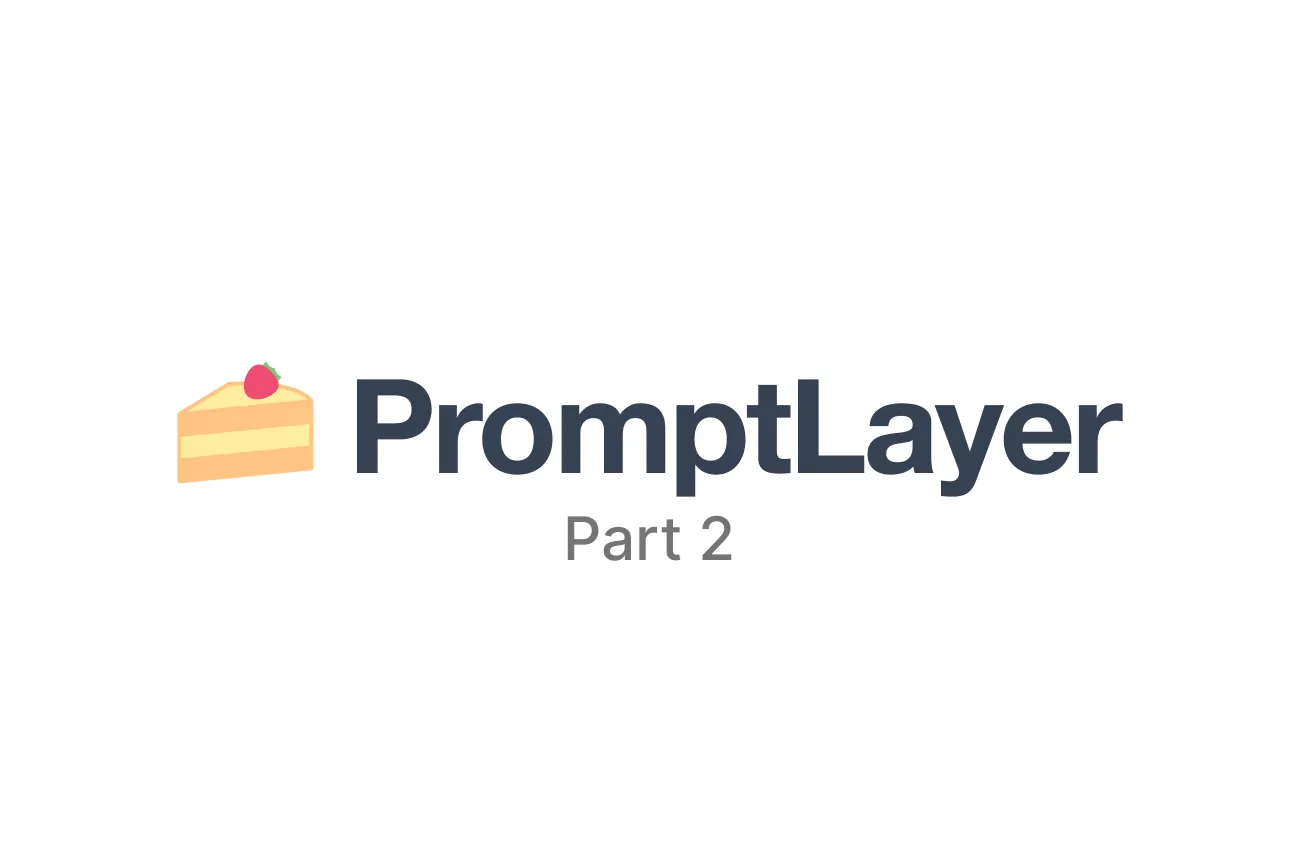
Introduction: Why Fine-Tune Prompts?
Crafting the perfect prompt is an art, but keeping it effective as requirements evolve is a science. Fine-tuning and evaluation are your secret weapons in prompt engineering. They ensure that your Large Language Models (LLMs) don’t just work—they work better over time.
In this article, we’ll dive deep into how PromptLayer simplifies fine-tuning and evaluations, empowering you to refine your prompts systematically. Whether you're a beginner experimenting with prompt tweaks or an advanced user optimizing for cost and latency, this guide has something for you.
What is Fine-Tuning in LLMs?
Fine-tuning is the process of tailoring a pre-trained LLM to your specific needs by training it on additional data. Why fine-tune?
- Reduce Costs: Train a cheaper model (e.g., GPT-3.5) using outputs from an expensive one (e.g., GPT-4).
- Improve Accuracy: Teach the model to handle specific tasks better.
- Save Tokens: Shorten prompts without degrading output quality.
- Streamline Output: Ensure responses match a desired format, like JSON.
Fine-tuning might sound complex, but with PromptLayer, it’s a breeze.
Step 1: Gather Training Data
Log Requests Automatically
The easiest way to gather training data is by logging LLM requests in PromptLayer. Simply wrap your OpenAI SDK as shown below:
from promptlayer import PromptLayer
promptlayer_client = PromptLayer(api_key="your_promptlayer_api_key")
OpenAI = promptlayer_client.openai.OpenAI
client = OpenAI()
response = client.chat.completions.create(
model="gpt-4",
messages=[
{"role": "system", "content": "You are a helpful assistant."},
{"role": "user", "content": "Explain quantum physics in simple terms."}
]
)
Each request and response will now appear in the PromptLayer Logs, creating a treasure trove of data for fine-tuning.
Generate Synthetic Data
Need a quick dataset? Use PromptLayer’s batch evaluation tools to run prompts against predefined test cases:
[
{"query": "Define artificial intelligence."},
{"query": "What is the capital of France?"}
]
Run these through GPT-4 to generate high-quality training data.
Step 2: Select and Export Data
Navigate to the Logs page on the PromptLayer dashboard to filter and export data for fine-tuning:
- Filters: Use tags, user IDs, or metadata to pinpoint relevant requests.
- Export: Download the filtered data as a JSON or CSV file for fine-tuning.
Here’s an example of selecting data programmatically:
promptlayer_client.track.metadata(
request_id=response["request_id"],
metadata={"user_id": "123", "label": "fine-tuning-data"}
)
Once you’ve gathered your data, you’re ready to fine-tune!
Step 3: Fine-Tune with PromptLayer
Kickstart Fine-Tuning
In the PromptLayer dashboard:
- Click the Fine-Tune button in the sidebar.
- Upload your training dataset.
- Select a base model (e.g.,
gpt-3.5-turbo
). - Configure parameters like learning rate, batch size, and epochs.
Monitor Progress
PromptLayer provides real-time updates on the fine-tuning process. You’ll see metrics like:
- Training loss
- Validation accuracy
- Cost
Once the job is complete, you’ll have a fine-tuned model hosted on OpenAI, ready for deployment!
Evaluations: The Key to Iteration
Fine-tuning is just half the battle. How do you ensure your new model actually works better? Enter evaluations.
What Are Evaluations?
Evaluations are systematic tests that measure a prompt’s performance. They help answer critical questions like:
- Does this prompt generate accurate results?
- How does the new version compare to the old one?
- Are edge cases being handled?
Step 1: Create a Dataset
Historical Data
You can create datasets from your PromptLayer logs:
- Filter requests by prompt template, version, or tag.
- Save the filtered set as a dataset for backtesting.
Upload Custom Data
You can also upload your own dataset in JSON or CSV format. For example:
JSON:
[
{"input": "What is 2+2?", "expected_output": "4"},
{"input": "Who wrote '1984'?", "expected_output": "George Orwell"}
]
CSV:
input,expected_output
"What is 2+2?","4"
"Who wrote '1984'?","George Orwell"
Step 2: Build an Evaluation Pipeline
An evaluation pipeline in PromptLayer is a series of steps to test your prompts systematically.
Example Pipeline
- Prompt Template: Run your prompt template using test cases.
- String Comparison: Compare outputs to expected results.
- LLM Assertion: Use an LLM to validate the quality of responses.
- Score Card: Aggregate results into a final score.
Configuring a Pipeline
Here’s an example of adding steps programmatically:
pipeline_config = {
"steps": [
{"type": "PROMPT_TEMPLATE", "template_name": "math_solver"},
{"type": "STRING_COMPARISON", "column_a": "output", "column_b": "expected_output"}
]
}
promptlayer_client.create_pipeline(pipeline_config)
Step 3: Run Evaluations
Once your pipeline is set up, run it against the dataset:
promptlayer_client.run_pipeline(pipeline_id="your_pipeline_id")
PromptLayer will generate detailed reports, including:
- Accuracy scores
- Visual diffs for mismatched outputs
- Cost and latency metrics
Step 4: Automate Continuous Integration
Integrate evaluations into your CI/CD pipeline. Every time you update a prompt or model, PromptLayer can automatically:
- Run evaluations
- Highlight regressions
- Score performance
Set this up by linking evaluation pipelines to your prompt templates.
Step 5: Analyze and Iterate
Use the Analytics page to track trends over time. Key insights include:
- Which prompts perform best
- Cost vs. accuracy trade-offs
- Common failure cases
Conclusion
Fine-tuning and evaluations are indispensable for taking your LLM applications from good to great. With PromptLayer, the process becomes intuitive and efficient:
- Log and collect training data.
- Fine-tune models with ease.
- Test prompts systematically with evaluations.
- Continuously improve based on real-world data.
PromptLayer equips you with the tools to iterate faster and smarter.
Until the next one,
Cohorte Team
February 26, 2025