Code Agents: The Swiss Army Knife of SmolAgents
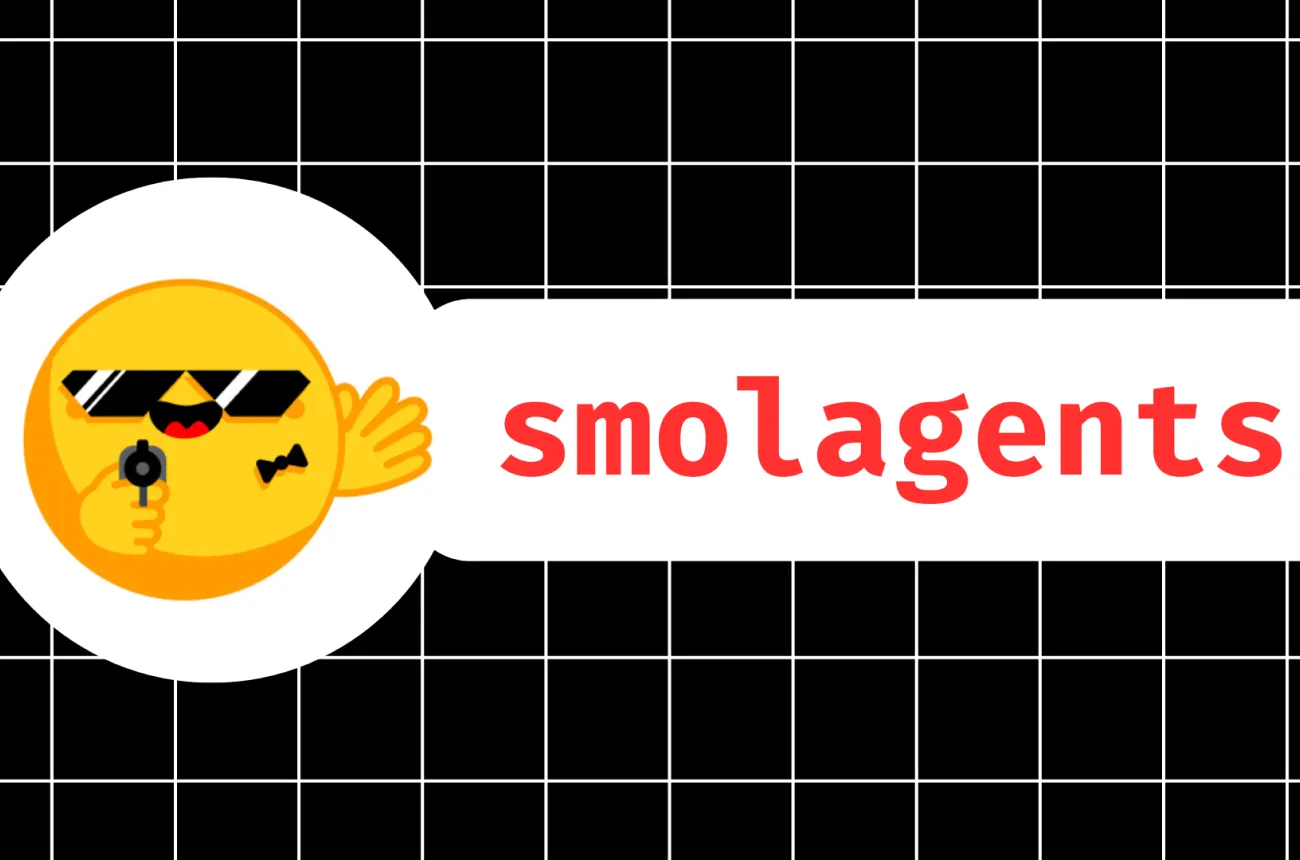
Introduction: Why Code Matters in Agentic Systems
Think about this: If you wanted to express every action you could perform on a computer, what would you use? Code, of course! Code is the universal language of computation. While JSON-based tool calling has been a popular standard in agentic systems, code agents—agents that generate and execute Python code—are proving to be a game-changer.
In this article, we’ll dive deep into Code Agents in SmolAgents, exploring how they work, their unique advantages, and how to use them effectively. Whether you’re a beginner or an advanced practitioner, this guide will take you step-by-step into the world of Code Agents.
1. What Are Code Agents?
A Code Agent is a special type of agent in SmolAgents that generates Python code snippets as part of its reasoning process. It doesn’t just stop at generating code—it executes it in a secure environment. This unlocks incredible flexibility and power for problem-solving.
Why Code Agents?
- Expressiveness: Python code is more flexible than JSON blobs, allowing nested logic, reusable functions, and complex workflows.
- Composability: Code naturally supports modular and reusable actions.
- Compatibility: Python has a rich ecosystem of libraries, making it easier to integrate new capabilities.
- LLM Familiarity: Modern LLMs are extensively trained on Python code, giving them a better understanding of how to write and debug it.
2. Setting Up Your First Code Agent
Let’s start with a simple example to build and run a Code Agent.
Step 1: Define the Model
The agent needs a text-generation model to power its reasoning. You can use any supported LLM.
python
Copy code
from smolagents import CodeAgent, HfApiModel
# Define the model
model = HfApiModel(model_id="meta-llama/Llama-3.3-70B-Instruct", token="<YOUR_HUGGINGFACE_API_TOKEN>")
Step 2: Create the Agent
Initialize the agent with the model and optional tools:
python
Copy code
agent = CodeAgent(tools=[], model=model, add_base_tools=True)
# Run a simple task
result = agent.run("What is the square root of 144?")
print(result)
3. How Code Agents Work
A Code Agent follows a Reason-Act-Observe cycle:
- Reason: The agent thinks about the problem and decides which tools or code snippets to use.
- Act: It generates Python code and executes it.
- Observe: It evaluates the results and adjusts its approach for the next step.
Here’s what happens under the hood:
- The agent generates Python code as output.
- The code is executed in a secure Python interpreter (or a sandboxed environment like E2B).
- The output of the execution is fed back into the agent for further reasoning.
4. Secure Code Execution
Executing arbitrary code can be risky. SmolAgents mitigates this with:
- Restricted Imports: Only safe modules (like
math
orjson
) are allowed. - Execution Limits: Infinite loops and resource-heavy operations are capped.
- Optional Sandboxing: Use the E2B Code Executor for maximum security.
Enabling E2B Execution
To enable sandboxed execution:
agent = CodeAgent(
tools=[],
model=model,
use_e2b_executor=True,
additional_authorized_imports=["requests"]
)
result = agent.run("Fetch the title of the webpage at https://huggingface.co/blog")
print(result)
5. Advanced Features of Code Agents
A. Using Tools with Code Agents
Code Agents can call tools in their generated code. For example:
from smolagents import tool
@tool
def add_numbers(a: int, b: int) -> int:
return a + b
agent = CodeAgent(tools=[add_numbers], model=model)
result = agent.run("Add 42 and 58.")
print(result)
B. Customizing Imports
By default, imports are restricted. You can add more:
agent = CodeAgent(
tools=[],
model=model,
additional_authorized_imports=["pandas"]
)
result = agent.run("Use pandas to create a DataFrame with 3 rows and 2 columns.")
print(result)
C. Debugging with Logs
Access detailed logs of the agent’s thought process:
agent.run("Calculate 2 + 2.")
print(agent.logs)
6. Real-World Applications
A. Data Analysis
Code Agents can perform advanced data analysis:
agent.run("Load the Titanic dataset from seaborn and calculate the survival rate.")
B. API Integration
Interact with APIs dynamically:
agent.run("Fetch the current weather in Paris using OpenWeatherMap API.")
C. Multi-Step Reasoning
Solve complex tasks step-by-step:
agent.run("First, find the square of 5. Then, multiply it by 3.")
7. Limitations and Best Practices
Limitations
- Model Quality: Performance depends on the underlying LLM.
- Error Handling: Generated code may fail if the task is ambiguous.
- Execution Time: Each step involves reasoning, generation, and execution.
Best Practices
- Provide Clear Prompts: Ambiguous tasks can lead to errors.
- Use Powerful LLMs: Choose a model trained extensively on Python.
- Test in Isolation: Test individual steps in complex workflows.
8. Multi-Agent Systems with Code Agents
Code Agents can collaborate with other agents in a multi-agent system. For example:
- A web search agent retrieves data.
- A code agent processes the data and performs calculations.
Here’s how to combine them:
from smolagents import ManagedAgent, DuckDuckGoSearchTool
web_agent = CodeAgent(tools=[DuckDuckGoSearchTool()], model=model)
managed_web_agent = ManagedAgent(agent=web_agent, name="web_search", description="Handles web searches.")
manager_agent = CodeAgent(
tools=[],
model=model,
managed_agents=[managed_web_agent]
)
result = manager_agent.run("Search for the tallest building in the world and calculate its height in miles.")
print(result)
9. Debugging Code Agents
A. Use a More Capable LLM
Switch to a more advanced model for complex tasks:
model = HfApiModel("Qwen/Qwen2.5-Coder-32B-Instruct")
B. Enhance System Prompts
Customize the agent’s system prompt for better guidance:
from smolagents.prompts import CODE_SYSTEM_PROMPT
custom_prompt = CODE_SYSTEM_PROMPT + "\nRemember to log every important step."
agent = CodeAgent(tools=[], model=model, system_prompt=custom_prompt)
C. Enable Planning
Let the agent plan its steps:
agent = CodeAgent(tools=[], model=model, planning_interval=3)
10. Conclusion: Why Code Agents Are the Future
Code Agents are not just tools—they’re a paradigm shift in how we think about agentic systems. By leveraging Python’s flexibility and the power of modern LLMs, they unlock possibilities that JSON-based agents simply cannot achieve.
Whether you’re automating workflows, analyzing data, or building multi-agent systems, Code Agents are your go-to solution for dynamic, real-world problem-solving.
Start building today and let your agents code their way to success. 🚀
For more in-depth information, visit the SmolAgents documentation.
Cohorte Team
February 20, 2025