Building Intelligent Chatbots with Azure Cognitive Services: A Complete Guide
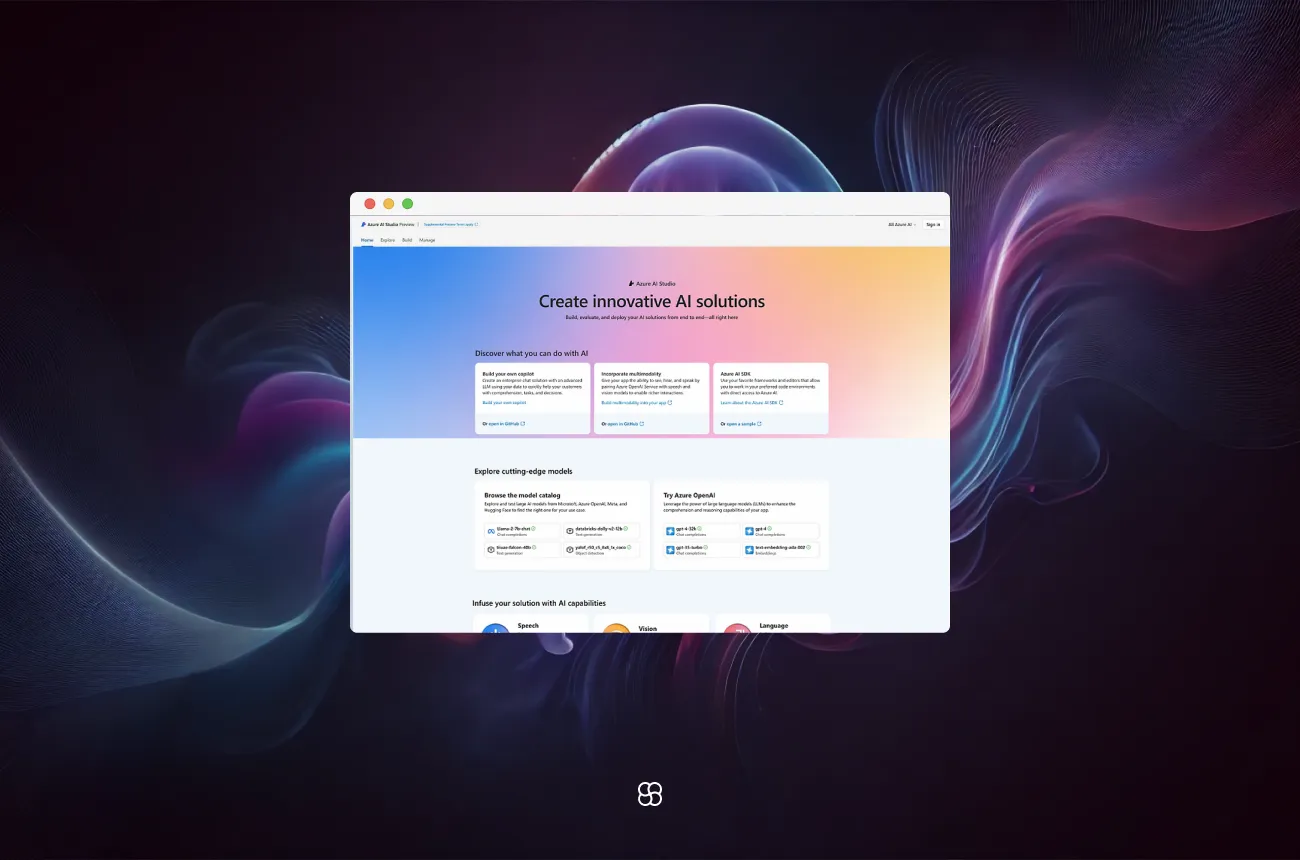
Building intelligent chatbots with Azure Cognitive Services enables developers to create sophisticated conversational agents that can understand and respond to user inputs effectively. This guide provides an in-depth, step-by-step approach to building a chatbot using Azure's suite of AI services, complete with code snippets and practical insights.
Presentation of the Framework
Azure Cognitive Services is a collection of AI services and APIs that allow developers to integrate intelligent features into their applications without extensive knowledge of AI or data science. These services include language understanding, speech recognition, vision processing, and more. In the context of chatbot development, Azure Cognitive Services, in conjunction with the Azure AI Bot Service, provides tools to create, test, deploy, and manage intelligent bots that can interact with users across various channels.
Key benefits
- Natural Language Understanding: Leverage Azure's Language Understanding (LUIS) to interpret user intents and entities, enabling more natural and intuitive interactions.
- Scalability: Azure's cloud infrastructure ensures that your chatbot can scale to handle a large number of users simultaneously.
- Multi-Channel Deployment: Deploy your chatbot across various platforms, including web, mobile, Microsoft Teams, and more, ensuring a consistent user experience.
- Integration with Other Services: Easily integrate with other Azure services and third-party APIs to enhance your chatbot's functionality.
Getting Started
Installation and Setup
- Azure Subscription: Ensure you have an active Azure subscription. If not, you can create a free account.
- Install Development Tools:
- Node.js: Download and install the latest version of Node.js.
- Bot Framework CLI: Install the Bot Framework Command Line Interface.
- Bot Framework CLI: Install the Bot Framework Command Line Interface.
- Set Up Azure Cognitive Services:
- In the Azure portal, create a new Language Understanding (LUIS) resource.
- Create a new QnA Maker resource if you plan to include FAQ capabilities.
First Steps
1. Create a New Bot Project:
npx @microsoft/generator-botbuilder
Follow the prompts to set up your bot project.
2. Configure LUIS:
- In your bot project, set up the LUIS application by providing the necessary keys and endpoint URLs.
- Define intents and entities in the LUIS portal to match user inputs appropriately.
3. Implement QnA Maker (Optional):
- Create a knowledge base in the QnA Maker portal.
- Populate it with question-and-answer pairs relevant to your application.
- Integrate the QnA Maker service into your bot by configuring the necessary keys and endpoints.
First Run
1. Start the Bot:
npm start
This command runs your bot locally.
2. Test with Bot Framework Emulator:
- Open the Bot Framework Emulator.
- Connect to your bot using the endpoint URL, typically
http://localhost:3978/api/messages
. - Interact with your bot to ensure it responds as expected.
Step-by-Step Example: Building a Simple FAQ Chatbot
Let's build a simple FAQ chatbot that uses LUIS for intent recognition and QnA Maker for answering frequently asked questions.
- Set Up LUIS:
- In the LUIS portal, create a new app named "FAQBot".
- Define an intent named "AskQuestion".
- Add example utterances that represent how users might ask questions.
- Train and publish the LUIS app.
- Set Up QnA Maker:
- In the QnA Maker portal, create a new knowledge base.
- Add question-and-answer pairs relevant to your FAQ.
- Save and publish the knowledge base.
- Integrate LUIS and QnA Maker into the Bot:
- In your bot's code, configure the LUIS and QnA Maker services by adding their keys and endpoints to your configuration file.
- Implement a dialog that uses LUIS to recognize the "AskQuestion" intent.
- When the "AskQuestion" intent is detected, forward the user's query to QnA Maker to retrieve the appropriate answer.
Sample Code:
const { LuisRecognizer, QnAMaker } = require('botbuilder-ai');
// LUIS configuration
const luisApplication = {
applicationId: process.env.LUIS_APP_ID,
endpointKey: process.env.LUIS_API_KEY,
endpoint: process.env.LUIS_ENDPOINT
};
const luisRecognizer = new LuisRecognizer(luisApplication);
// QnA Maker configuration
const qnaMaker = new QnAMaker({
knowledgeBaseId: process.env.QNA_KB_ID,
endpointKey: process.env.QNA_ENDPOINT_KEY,
host: process.env.QNA_HOST
});
// Bot logic
this.onMessage(async (context, next) => {
const luisResult = await luisRecognizer.recognize(context);
const intent = LuisRecognizer.topIntent(luisResult);
if (intent === 'AskQuestion') {
const qnaResults = await qnaMaker.getAnswers(context);
if (qnaResults.length > 0) {
await context.sendActivity(qnaResults[0].answer);
} else {
await context.sendActivity('I\'m sorry, I don\'t have an answer for that.');
}
} else {
await context.sendActivity('I\'m sorry, I didn\'t understand that.');
}
await next();
});
This code sets up the LUIS and QnA Maker services and defines the bot's behavior when it receives a message.
- Test the Bot:
- Run your bot locally.
- Use the Bot Framework Emulator to interact with your bot.
- Ask questions that are in your QnA Maker knowledge base to see if the bot responds correctly.
- Test phrases that should trigger the "AskQuestion" intent in LUIS to ensure proper intent recognition.
- Deploy the Bot:
- Once you're satisfied with the bot's performance, deploy it to Azure.
- In the Azure portal, create a new Web App Bot resource.
- Configure the deployment settings and publish your bot.
- After deployment, test the bot using the Web Chat feature in the Azure portal.
- Integrate with Channels:
- In the Azure portal, navigate to your bot's resource.
- Under "Bot Management," select "Channels."
- Add the desired channels (e.g., Microsoft Teams, Slack, Facebook Messenger) to make your bot available to users on those platforms.
Final Thoughts
Building intelligent chatbots with Azure Cognitive Services empowers developers to deliver scalable, versatile, and engaging user experiences. By integrating services like LUIS and QnA Maker, your chatbot can understand user intents and provide relevant, accurate responses. Azure’s flexible deployment options and seamless integrations make it the perfect platform for developing powerful conversational agents.
Further Learning
Start building smarter chatbots today with Azure Cognitive Services and deliver conversations that matter!
Cohorte Team
January 17, 2025