Building Context-Aware Chatbots: A Step-by-Step Guide Using LlamaIndex
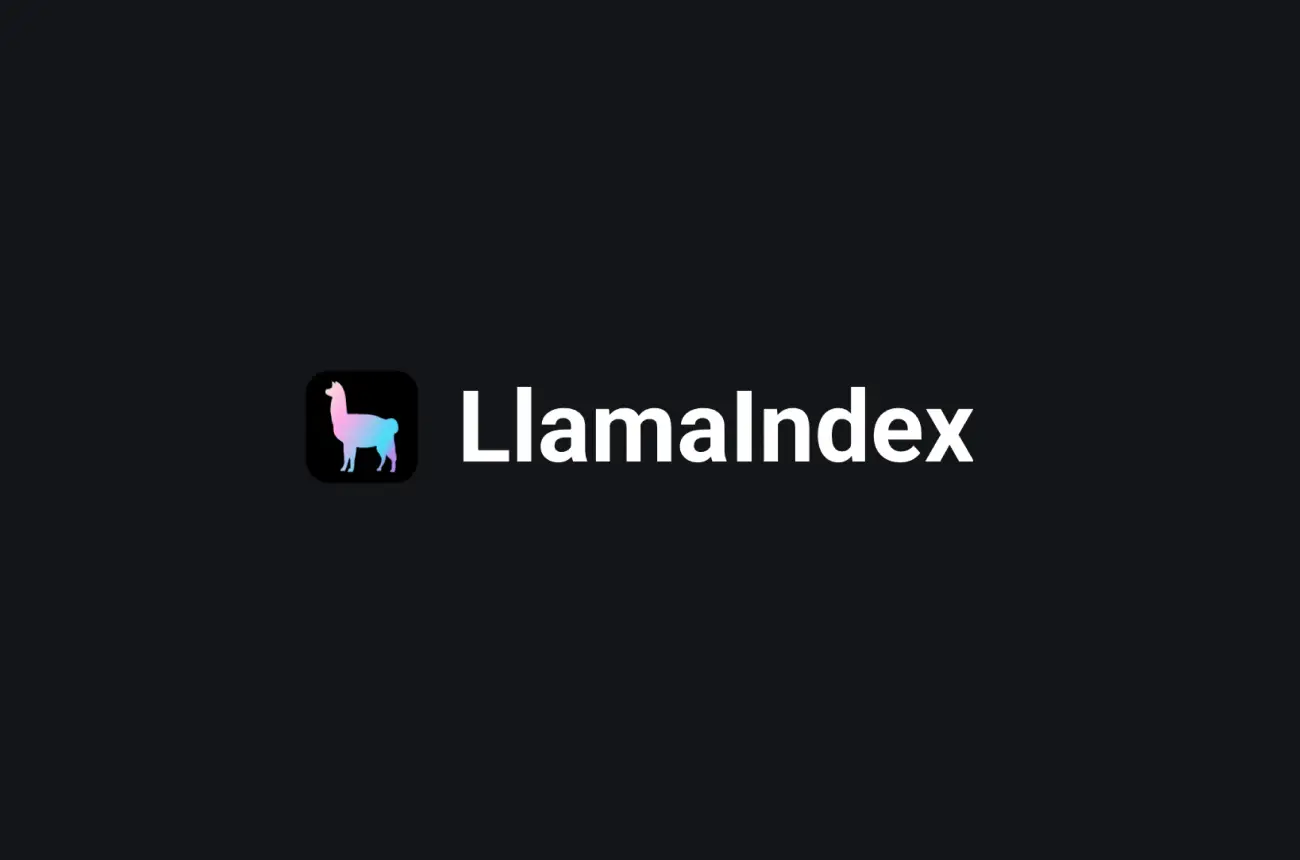
Building context-aware chatbots enhances user interactions by providing relevant and accurate responses based on the conversation's context. LlamaIndex is a powerful data framework that connects Large Language Models (LLMs) with external data sources, enabling the development of intelligent, context-augmented chatbots. This guide will walk you through understanding LlamaIndex, its benefits, and a step-by-step process to build a simple context-aware chatbot.
Understanding LlamaIndex
LlamaIndex serves as a bridge between your data and LLMs, providing a toolkit that enables you to establish a query interface around your data for various tasks, such as question-answering and summarization. It allows developers to create chatbots that can intelligently execute tasks over their data, resulting in more informed and contextually relevant interactions.
Key Benefits:
- Contextual Understanding: Enhances chatbots' ability to retain and utilize conversation context, leading to more coherent and user-friendly interactions.
- Efficient Data Retrieval: Manages large datasets, enabling chatbots to pull specific, relevant information, thereby providing accurate answers.
- Scalability: Suitable for applications handling numerous queries, making it ideal for organizations with high-volume interactions.
- Customizability: Offers a high level of customization, allowing developers to tailor chatbots to specific use cases.
Getting Started with LlamaIndex
1. Installation and Setup:
- Prerequisites:
- Python 3.7 or higher.
- An OpenAI API key.
- Installation:Install the necessary packages:
pip install llama-index openai
- Set Up API Key:Configure your OpenAI API key:
import os
os.environ["OPENAI_API_KEY"] = "your_openai_api_key"
2. Ingesting Data:
- Prepare Your Data:Gather the documents or data sources you want your chatbot to access.
- Load and Process Data:Use LlamaIndex to load and process your data:
from llama_index import SimpleDirectoryReader, GPTVectorStoreIndex
# Load documents from a directory
documents = SimpleDirectoryReader('path_to_your_data').load_data()
# Create an index
index = GPTVectorStoreIndex.from_documents(documents)
3. Building the Chatbot:
- Set Up the Chat Engine:Configure the chat engine to handle user interactions:
from llama_index import ChatEngine
# Initialize the chat engine
chat_engine = ChatEngine.from_index(index)
- Implement the Chat Interface:Create a simple loop to handle user input and generate responses:
while True:
user_input = input("You: ")
if user_input.lower() == "exit":
break
response = chat_engine.chat(user_input)
print(f"Bot: {response}")
Step-by-Step Example: Building a Simple Context-Aware Chatbot
1. Install Required Libraries:
Ensure you have the necessary libraries installed:
pip install llama-index openai
2. Set Up Your Environment:
Configure your OpenAI API key:
import os
os.environ["OPENAI_API_KEY"] = "your_openai_api_key"
3. Prepare Your Data:
Organize your documents in a directory (e.g., 'data/') for the chatbot to access.
4. Load and Index the Data:
Use LlamaIndex to load and index your documents:
from llama_index import SimpleDirectoryReader, GPTVectorStoreIndex
# Load documents from the 'data' directory
documents = SimpleDirectoryReader('data').load_data()
# Create an index from the documents
index = GPTVectorStoreIndex.from_documents(documents)
5. Initialize the Chat Engine:
Set up the chat engine using the created index:
from llama_index import ChatEngine
# Initialize the chat engine
chat_engine = ChatEngine.from_index(index)
6. Create the Chat Interface:
Implement a simple loop to handle user input and generate responses:
while True:
user_input = input("You: ")
if user_input.lower() == "exit":
break
response = chat_engine.chat(user_input)
print(f"Bot: {response}")
7. Run the Chatbot:
Execute your script and interact with the chatbot:
python your_script.py
Type your questions, and the chatbot will provide contextually relevant responses based on the ingested data.
Final Thoughts
By leveraging LlamaIndex, developers can create sophisticated, context-aware chatbots that provide users with accurate and relevant information. The framework's ability to integrate external data sources with LLMs enhances the chatbot's understanding and responsiveness, leading to more meaningful user interactions. As AI continues to evolve, tools like LlamaIndex will play a crucial role in developing intelligent applications that can effectively manage and utilize vast amounts of data.
Cohorte Team
February 7, 2025