Building a Role-Based AI Development Team with the OpenAI Agent SDK
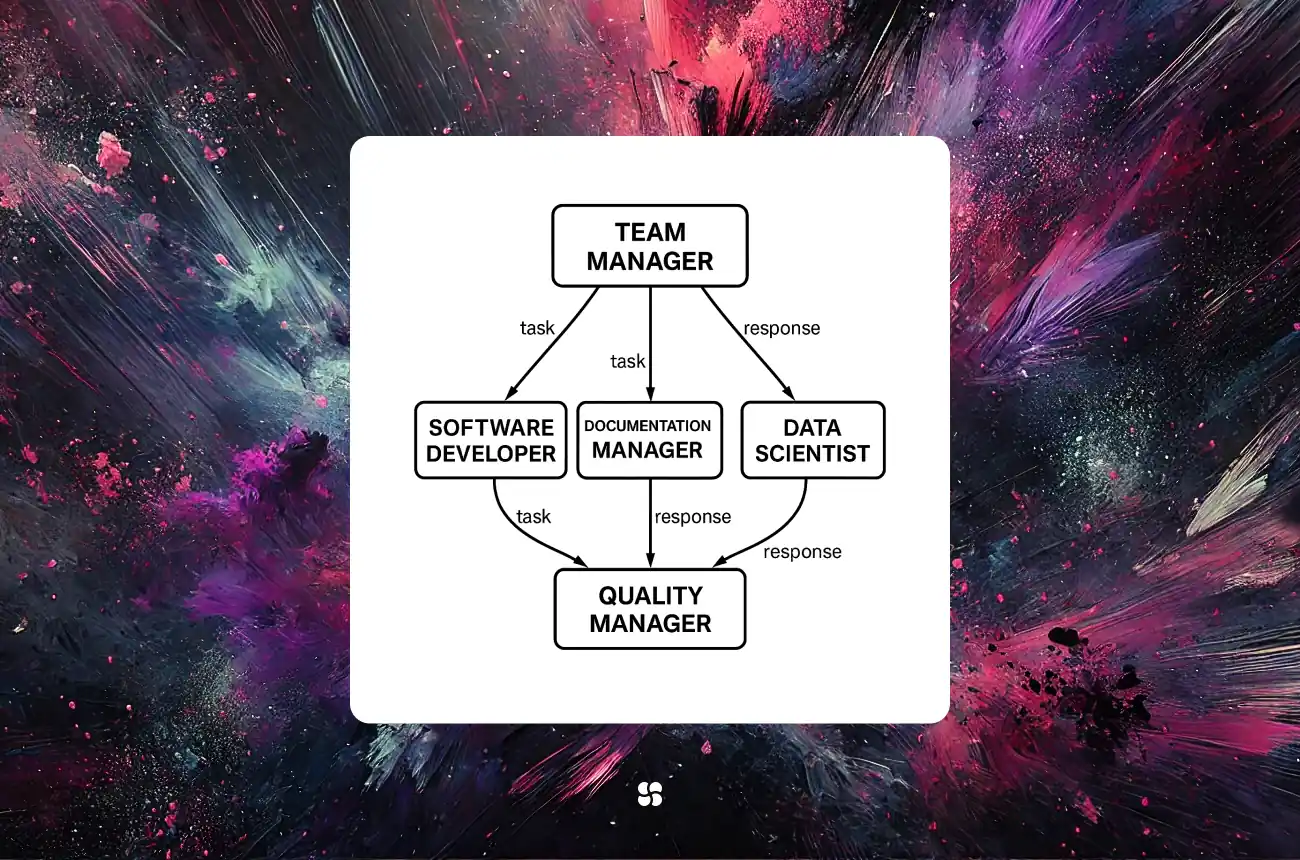
AI Agents are eating software. With the OpenAI Agent SDK, you can not only automate many mundane tasks, but also orchestrate a team of agents that handle code development, documentation, data science, and quality assurance. This deep dive will walk you through each part of the journey—from understanding the framework to deploying a full-fledged agent-based team.
1. Presentation of the Framework
The OpenAI Agent SDK provides a modular platform for creating agents that can reason, generate code, and collaborate on complex projects. At its core, the SDK abstracts many foundational programming tasks, allowing you to define agents using simple classes and functions that interact through well-defined protocols. Key components include:
- Agent Definition and Lifecycle: Each agent encapsulates responsibilities specific to a role and can be extended with custom logic.
- Task Coordination: The framework supports passing tasks and data between agents, ensuring that the right expertise is applied to each problem.
- Communication Protocols: Standardized message formats and callback mechanisms allow agents to collaborate seamlessly.
- Extensibility: The SDK’s architecture makes it straightforward to add or modify the behavior of agents, turning them into specialized units (e.g., coders, data analyzers, documenters).
For more information on these foundational elements, please refer to the OpenAI Agent SDK documentation.
2. Defining the Roles: Team Components
A robust development process benefits from role-specific agents. Here’s a breakdown of the roles we’re integrating:
- Team Manager & Software Developer:
– Responsibilities: Oversees the project, delegates tasks, and implements core logic for dynamic team management.
– Value: Ensures that coding and coordination strategies are implemented effectively. - Documentation Manager:
– Responsibilities: Manages project documentation, updates readmes, and tracks code changes in a clear and concise manner.
– Value: Guarantees that all team actions are logged and accessible, improving maintainability. - Data Scientist:
– Responsibilities: Analyzes project data, builds models to predict problem areas, and optimizes workflows through data insights.
– Value: Improves decision-making and drives data-informed development strategies. - Quality Manager:
– Responsibilities: Oversees testing protocols, ensures code quality, conducts reviews, and integrates automated testing.
– Value: Maintains a high standard of software reliability and performance.
Each of these roles can be modeled as distinct agents that work in concert via the OpenAI Agent SDK.
3. Orchestrating the Agents: How the Team Manager Works
The Team Manager is the heartbeat of this system.
It receives and analyzes tasks, identifies the task type, and routes each task using specific agent prompts.
Here’s how the orchestration unfolds:
Dynamic Task Routing
- Task Analysis:
The manager inspects the properties of every incoming task (such as its type, name, and description). - Agent Selection:
Based on the task type—development, documentation, data, or quality—it delegates work to the corresponding agent. - Response Aggregation:
After an agent completes its task, the Team Manager gathers responses to provide a comprehensive outcome.
This process is implemented using the agent’s built-in respond
methods, ensuring each specialized prompt guides behavior.
4. Agent Prompts Using the OpenAI Agent SDK
In an SDK environment, agents are instantiated with role-specific prompts rather than simple Python classes.
Below are sample definitions for each agent:
from openai_agents import Agent
# Software Developer Agent
software_agent = Agent(
name="Software Developer",
prompt="You are an experienced software developer. Write efficient and clean code to solve the problem. Follow best practices and include useful comments."
)
# Documentation Manager Agent
documentation_agent = Agent(
name="Documentation Manager",
prompt="You are a meticulous documentation manager. Update and maintain project documentation in clear, concise language, ensuring accuracy and ease of use."
)
# Data Scientist Agent
data_scientist_agent = Agent(
name="Data Scientist",
prompt="You are a data scientist. Analyze the provided data, generate insights, and suggest improvements based on statistical analysis and performance metrics."
)
# Quality Manager Agent
quality_agent = Agent(
name="Quality Manager",
prompt="You are a quality manager. Verify that all code adheres to high standards, execute automated tests, and provide clear feedback on any issues or improvements."
)
# Team Manager Agent – Orchestrates the other agents
team_manager = Agent(
name="Team Manager",
prompt=("You are the project overseer. For each task, determine whether it is a development, documentation, data analysis, or quality assurance issue. "
"Delegate it to the corresponding agent. Then, aggregate the outcomes into a comprehensive report.")
)
5. Step-by-Step Guide to Building Your Agent Team
Step 1: Environment Setup
Begin by installing the OpenAI Agent SDK. If you haven’t already installed it, you can typically do so using pip:
pip install openai-agents
Ensure your development environment is configured with the necessary API credentials and access tokens for smooth integration with OpenAI services.
Step 2: Define Agent Prompts
Use the above code snippet to instantiate your agents with clear, role-specific prompts.
Step 3: Orchestrate with the Team Manager
Create a function that uses the Team Manager to analyze and delegate tasks:
def orchestrate_task(task):
task_type = task.get("type")
if task_type == "development":
return software_agent.respond(task)
elif task_type == "documentation":
return documentation_agent.respond(task)
elif task_type == "data":
return data_scientist_agent.respond(task)
elif task_type == "quality":
return quality_agent.respond(task)
else:
return {"status": "error", "message": "Unknown task type"}
# Example task submission
task = {
"name": "Implement Feature X",
"type": "development",
"description": "Develop a new feature with an emphasis on performance and modularity."
}
# The Team Manager analyzes and delegates the task
result = orchestrate_task(task)
print(result)
Step 4: Monitoring and Iterative Improvement
- Logging:
Each agent logs its progress and outcomes. - Error Handling:
Build in robust error detection for smoother operations. - Iterate:
Use data insights from the Data Scientist to refine processes continuously.
6. Final Thoughts
Building a team of agent is becoming easier with the OpenAI Agent SDK. You just ned to create the structure connect the agents, and you can have something running quickly in a few minutes.
For deeper details on the OpenAI Agent SDK and its capabilities, please refer to the official documentation .
Cohorte Team
April 10, 2025