Building a Financial Analysis Assistant with LlamaIndex and Streamlit
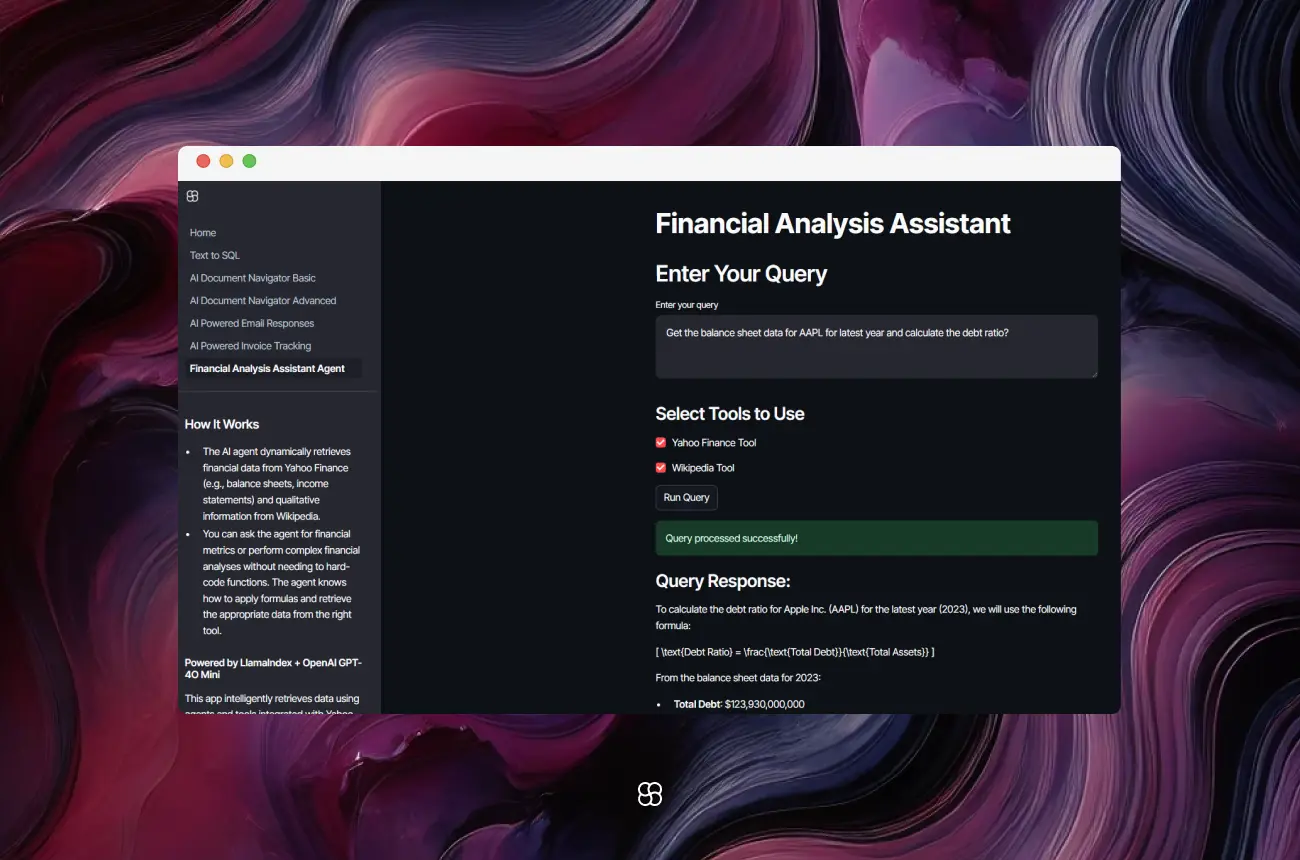
Introducing our latest project: a Financial Analysis Assistant that simplifies data crunching. If you're looking for a smarter way to analyze financial statements without the spreadsheet overload, this is your project.
Introducing Our Financial Analysis Assistant Agent
So, what's this all about? We've developed a handy agent based app that acts like your personal financial analyst. You can ask it questions like:
- "Get the balance sheet data for AAPL for the latest year and calculate the debt ratio."
- "What is the revenue growth rate for Google over the past year?"
And it will fetch the data, perform the calculations, and provide you with clear answers—all without you having to write a single formula. Pretty neat, right?
How Does It Work?
Under the hood, our assistant leverages:
- LlamaIndex Agent and Tools: These agents intelligently decides which tool to use to fetch the data and how to process it.
- OpenAI GPT-4O Mini: The language model that understands your queries and helps generate insightful responses.
- Yahoo Finance and Wikipedia Integration: For accessing the latest financial data and company information.
Let's Dive Into the Code
Let's take a closer look at how we've put this together.
Setting Up the App with Streamlit
First off, we're using Streamlit to build the web app. It's a fantastic framework that turns Python scripts into interactive web applications.
import streamlit as st
st.title("Financial Analysis Assistant")
Handling User Input
We capture the user's query—the question they want the assistant to answer.
st.header("Enter Your Query")
query = st.text_area(
"Enter your query",
"Get the balance sheet data for AAPL for the latest year and calculate the debt ratio?"
)
Tool Selection
We allow users to choose which tools they want to use. They might only need financial data from Yahoo Finance, or perhaps they want to include information from Wikipedia as well.
st.subheader("Select Tools to Use")
use_yfinance = st.checkbox("Yahoo Finance Tool", True)
use_wikipedia = st.checkbox("Wikipedia Tool", False)
Running the Query
When the user clicks "Run Query", here's what happens:
if st.button("Run Query"):
if not api_key:
st.error("Please provide an OpenAI API key to continue.")
else:
# Load the OpenAI language model
llm = OpenAI(model="gpt-4o-mini", api_key=api_key)
# Initialize tools based on the user's selection
combined_tools = []
if use_yfinance:
finance_tool = YahooFinanceToolSpec()
combined_tools += finance_tool.to_tool_list()
if use_wikipedia:
wiki_tool = WikipediaToolSpec()
combined_tools += wiki_tool.to_tool_list()
# Ensure at least one tool is selected
if not combined_tools:
st.error("No tools selected. Please select at least one tool to proceed.")
else:
# Create the agent
agent = OpenAIAgent.from_tools(combined_tools, llm=llm)
# Process the query
with st.spinner("Processing your query..."):
try:
response = agent.chat(query)
st.success("Query processed successfully!")
st.write(f"### Query Response:\n\n{response}")
except Exception as e:
st.error(f"Error: {str(e)}")
What's Happening Here?
- API Key Check: We verify that the API key is provided.
- Tool Initialization: Based on the user's selection, we set up the appropriate tools.
- Agent Creation: We instantiate an agent that knows how to use these tools.
- Query Processing: The agent processes the user's query and returns the response.
Example: Calculating the Debt Ratio
Let's say you ask for the debt ratio for Apple (AAPL). Here's the step-by-step process:
- Data Retrieval: The agent fetches the latest balance sheet data for AAPL from Yahoo Finance.
- Calculation: It knows the formula for the debt ratio (Total Debt / Total Assets) and performs the calculation using the retrieved data.
- Response Generation: It presents the result in a clear and understandable format.
How the Agent Works
Our agent is designed to be intelligent and efficient:
- Understands Natural Language: Thanks to OpenAI's GPT-4O Mini, it comprehends complex financial queries.
- Intelligent Tool Selection: It autonomously chooses the right tool—Yahoo Finance for detailed financials or Wikipedia for broader company info—based on your query.
- Dynamic Data Processing: It applies financial formulas on-the-fly without the need for hard-coded functions.
Code Snippets for the Agent and Tools
Here's how we set up the language model and tools:
from llama_index.agent.openai import OpenAIAgent
from llama_index.llms.openai import OpenAI
from llama_index.tools.yahoo_finance import YahooFinanceToolSpec
from llama_index.tools.wikipedia import WikipediaToolSpec
# Load the OpenAI language model
llm = OpenAI(model="gpt-4o-mini", api_key=api_key)
# Initialize tools
combined_tools = []
if use_yfinance:
finance_tool = YahooFinanceToolSpec()
combined_tools += finance_tool.to_tool_list()
if use_wikipedia:
wiki_tool = WikipediaToolSpec()
combined_tools += wiki_tool.to_tool_list()
# Create the agent
agent = OpenAIAgent.from_tools(combined_tools, llm=llm)
Enhancing the User Interface
We wanted the app to be visually appealing, so we added some custom CSS styling.
with open('style.css') as css:
st.markdown(f'<style>{css.read()}</style>', unsafe_allow_html=True)
Adding Helpful Information in the Sidebar
To make the app more user-friendly, we've included a sidebar that explains how the app works and acknowledges the technologies powering it.
st.sidebar.header("How It Works")
st.sidebar.write("""
- The AI agent dynamically retrieves financial data from Yahoo Finance (e.g., balance sheets, income statements) and qualitative information from Wikipedia.
- You can ask the agent for financial metrics or perform complex financial analyses without needing to hard-code functions. The agent knows how to apply formulas and retrieve the appropriate data from the right tool.
""")
st.sidebar.write("""
#### Powered by LlamaIndex + OpenAI GPT-4O Mini
This app intelligently retrieves data using agents and tools integrated with Yahoo Finance and Wikipedia to assist you in your financial research.
""")
Why This Is Awesome
- Eliminates Manual Calculations: No need to manually search for data or perform complex calculations.
- Smart Data Retrieval: The agent knows exactly where to find the information you need.
- Flexible and Scalable: Easily add more tools or analytical capabilities without extensive reprogramming.
- User-Friendly Experience: Accessible to both novice and expert users for a wide range of financial research needs.
Wrapping Up
Building this Financial Analysis Assistant Agent has been a great experience for us. We're really excited about how it can make financial data analysis easier and more accessible. We're looking forward to adding more features and seeing how it helps you with your financial research.
Author
.png)