Automating Document Analysis with Azure AI Document Intelligence: A Comprehensive Step-by-Step Guide
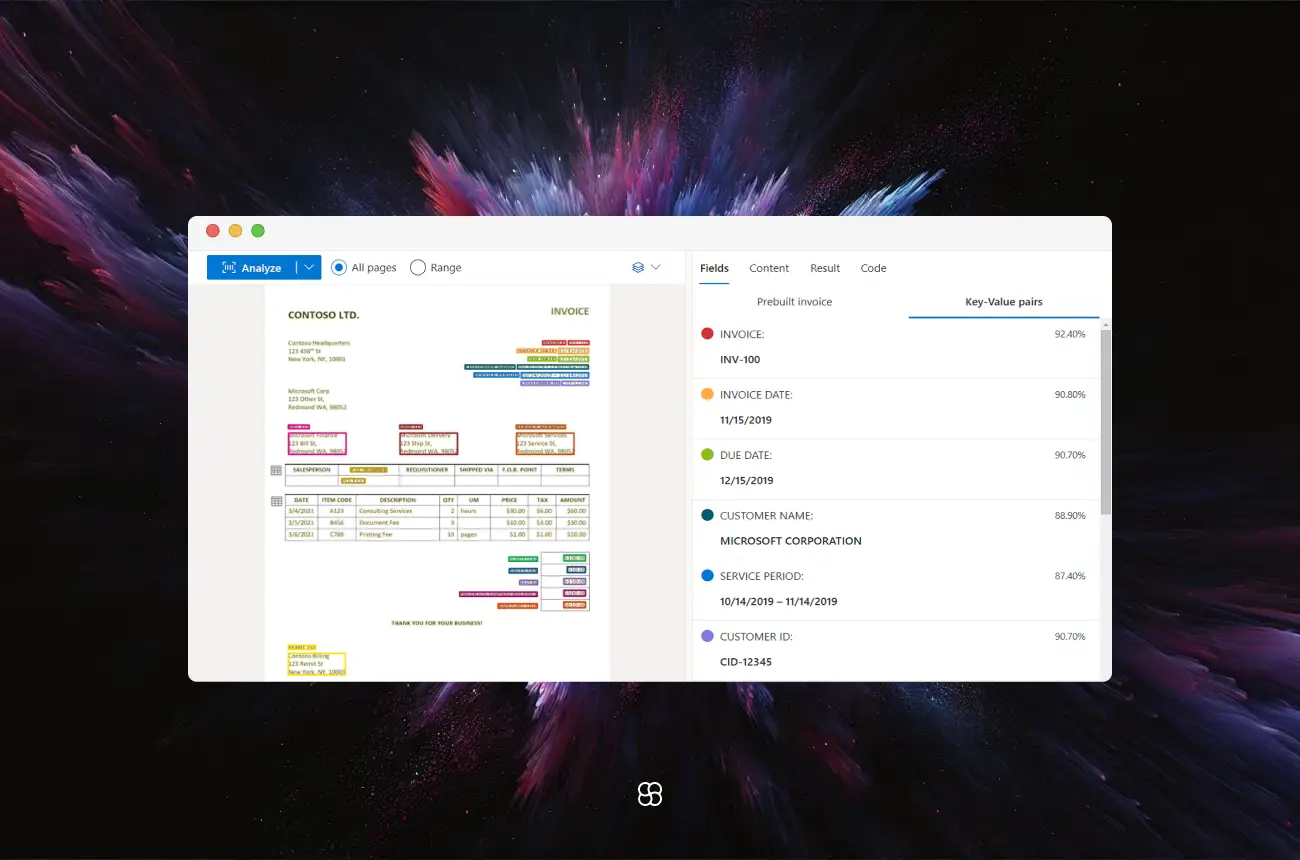
Automating document analysis is essential for enhancing efficiency and accuracy in data processing workflows. Azure AI Document Intelligence, formerly known as Form Recognizer, offers robust capabilities for extracting text, key-value pairs, tables, and structures from various document types. This guide provides a comprehensive approach to utilizing Azure AI Document Intelligence for automated document analysis, complete with code snippets and practical insights.
Presentation of Azure AI Document Intelligence
Azure AI Document Intelligence is a cloud-based service that applies advanced machine learning to extract information from documents. It supports a wide range of document formats, including PDFs, images (JPEG, PNG, TIFF), and Office documents (Word, Excel, PowerPoint). The service offers pre-built models for common document types like invoices, receipts, and business cards, as well as custom models that can be trained to recognize specific fields and formats unique to your business needs.
Benefits
- Efficiency: Automates the extraction of data from documents, reducing manual effort and processing time.
- Accuracy: Utilizes machine learning models to accurately extract structured data, minimizing errors associated with manual data entry.
- Scalability: Handles large volumes of documents, making it suitable for enterprises with extensive data processing requirements.
- Customization: Allows training of custom models to cater to specific document formats and business needs.
Getting Started
Installation and Setup
- Azure Subscription: Ensure you have an active Azure subscription. If not, you can create a free account.
- Create a Document Intelligence Resource:
- Navigate to the Azure portal.
- Click on "Create a resource" and search for "Azure AI Document Intelligence".
- Follow the prompts to create the resource, selecting the appropriate subscription, resource group, and region.
- Obtain API Key and Endpoint:
- After creating the resource, go to the resource's "Keys and Endpoint" section.
- Note the API key and endpoint URL; these will be used for authentication in your application.
- Install Required Packages:
- Ensure you have Python installed.
- Install the Azure AI Document Intelligence client library:
pip install azure-ai-formrecognizer
First Steps
1. Import Libraries:
from azure.ai.formrecognizer import DocumentAnalysisClient
from azure.core.credentials import AzureKeyCredential
2. Initialize the Client:
endpoint = "YOUR_FORM_RECOGNIZER_ENDPOINT"
api_key = "YOUR_FORM_RECOGNIZER_KEY"
client = DocumentAnalysisClient(
endpoint=endpoint,
credential=AzureKeyCredential(api_key)
)
3. Analyze a Document:
with open("path_to_your_document.pdf", "rb") as f:
poller = client.begin_analyze_document("prebuilt-document", document=f)
result = poller.result()
Advanced Features and Applications
Custom Model Training
While pre-built models are suitable for common document types, Azure AI Document Intelligence allows you to train custom models tailored to your specific documents. This is particularly useful for forms or documents with unique layouts or fields.
- Data Collection:
- Gather a diverse set of documents representative of the variations you expect.
- Ensure each document is accurately labeled with the fields you wish to extract.
- Upload Training Data:
- Store your training documents in an Azure Blob Storage container.
- Generate a Shared Access Signature (SAS) token for secure access.
- Train the Custom Model:
- Use the Azure AI Document Intelligence Studio or SDK to initiate training.
- Specify the storage container and SAS token.
- Monitor the training process and validate the model upon completion.
For detailed guidance, refer to the official documentation.
Custom Classification Models
In scenarios where you need to identify the document type before extracting data, Azure AI Document Intelligence supports custom classification models. These models can categorize documents, enabling workflows that require different processing based on document type.
- Prepare Training Data:
- Collect labeled examples for each document category.
- Ensure diversity in the training set to capture variations within each category.
- Train the Classifier:
- Use the Azure AI Document Intelligence Studio or SDK to train the classifier.
- Provide the labeled data and configure training parameters.
- Integrate into Workflow:
- Deploy the classifier to automatically route documents to appropriate processing pipelines based on their type.
Detailed instructions are available in the documentation.
Building a Simple Document Analysis Agent
Let's build a simple agent that extracts text and tables from a document and prints the results.
1. Define the Function to Analyze Documents:
def analyze_document(file_path):
with open(file_path, "rb") as f:
poller = client.begin_analyze_document("prebuilt-document", document=f)
result = poller.result()
return result
2. Extract and Print Content:
def extract_content(result):
# Extract text
print("----Extracted Text----")
for page in result.pages:
for line in page.lines:
print(line.content)
# Extract tables
print("----Extracted Tables----")
for table in result.tables:
for cell in table.cells:
print(f"Cell[{cell.row_index}][{cell.column_index}]: {cell.content}")
This function iterates through the analyzed result to print extracted text and table data.
3. Main Function:
if __name__ == "__main__":
file_path = "path_to_your_document.pdf"
result = analyze_document(file_path)
extract_content(result)
Replace "path_to_your_document.pdf"
with the actual path to your document. Running this script will display the extracted text and table content in the console.
Advanced Applications
Beyond basic text and table extraction, Azure AI Document Intelligence offers advanced capabilities:
- Custom Model Training: Train models to recognize specific fields and formats unique to your business documents. This is particularly useful for forms and documents with unique layouts.
- Document Classification: Automatically classify documents into predefined categories, streamlining document management workflows.
- Integration with Business Processes: Incorporate document analysis into larger workflows, such as automated invoice processing, contract management, and data entry automation.
Final Thoughts
Azure AI Document Intelligence provides a powerful platform for automating document analysis, enhancing efficiency, accuracy, and scalability in data processing tasks. By leveraging its capabilities, businesses can streamline operations, reduce manual errors, and focus on higher-value activities.
For more detailed information and advanced use cases, refer to the Azure AI Document Intelligence client library for Python documentation.
Additionally, explore the Azure Document Intelligence code samples for practical examples and further guidance.
By integrating Azure AI Document Intelligence into your workflows, you can achieve significant improvements in document processing efficiency and accuracy.
Cohorte Team
January 16, 2025