Agentic AI: Getting Started Guides with Frameworks
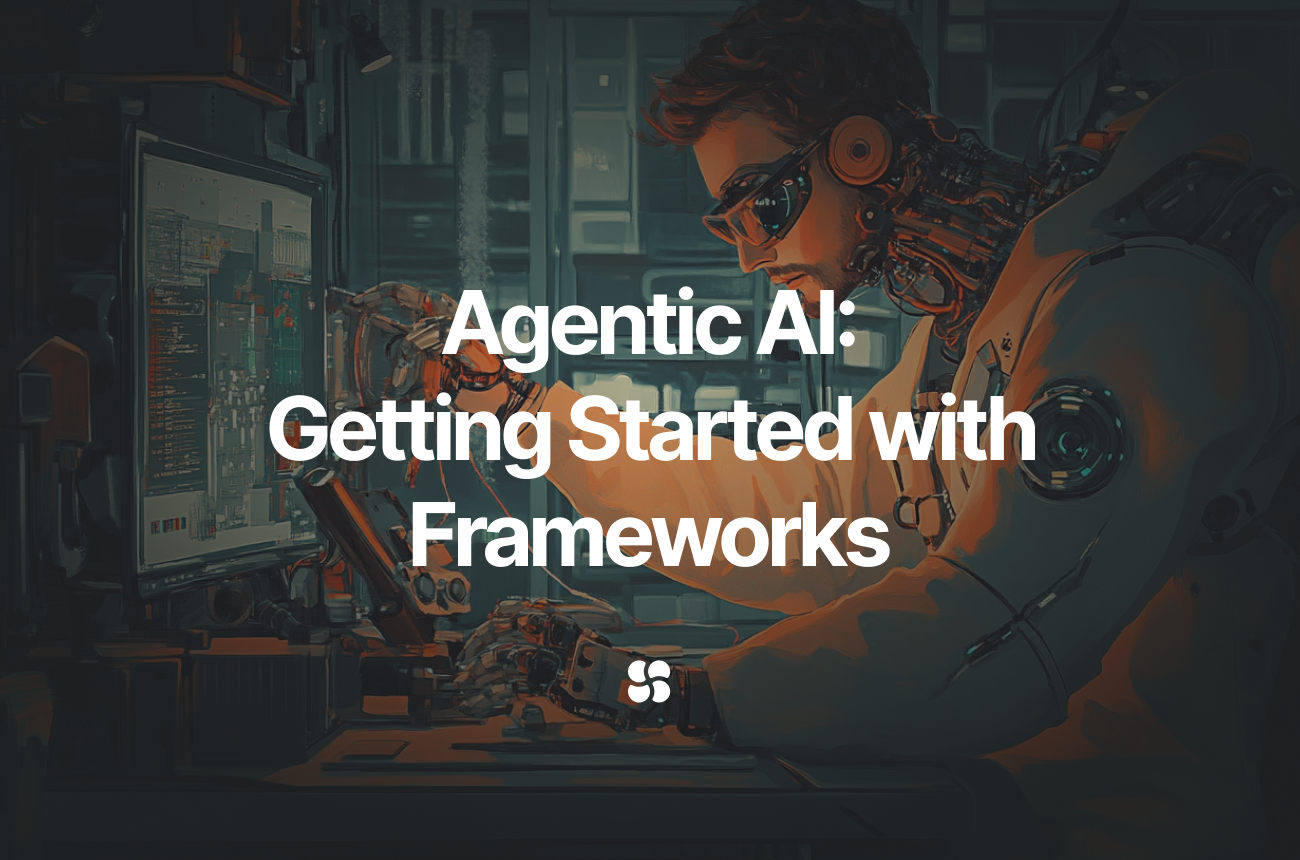
Implementing an agentic AI from scratch can be complex, but thankfully several frameworks have emerged to simplify the process. These frameworks provide pre-built modules for things like connecting to language models, managing conversation memory, defining tools, and orchestrating multi-step reasoning. In this article, we’ll give an overview of five popular frameworks for building AI agents – LangChain, LangGraph, LlamaIndex, CrewAI, and Hugging Face’s SmolAgent – and guide you on how to get started with each. We’ll cover how to set up your environment, core concepts of each framework, and tips on choosing the right tool for your needs. By the end, you should have a clear idea of how to spin up a basic agent in Python with these libraries.
Before diving in, ensure you have a Python 3.x environment ready and API keys for any LLMs you plan to use (e.g., OpenAI API key or others), since these frameworks typically interface with LLM providers.
LangChain
What it is: LangChain is one of the most widely-used frameworks for developing applications powered by LLMs. It provides abstractions for chains (sequences of prompts/actions), agents (LLM-powered decision makers with tool use), memory modules, and a vast array of integrations with external services. LangChain’s philosophy is to simplify every stage of the LLM app lifecycle – from development and prompt management to evaluation and deployment. For agentic AI, LangChain offers high-level classes to create agents that can use tools, along with many ready-made tool integrations (web search, math, Wikipedia, etc.).
Setup: You can install LangChain via pip. For example, to include OpenAI model support and basic tools:
pip install langchain openai
(LangChain is modular; you might install specific extras like langchain[serpapi]
for web search or others as needed.)
Make sure to set your API keys (e.g., OPENAI_API_KEY
) as environment variables or in code before using LLMs.
Core Concepts: In LangChain, an Agent is essentially an LLM plus a set of Tools. One common pattern is the ReACT agent which uses the ReAct loop we described. LangChain provides an AgentExecutor
that takes an LLM and tools and handles the loop of prompts and tool calls for you. There are different agent types (zero-shot react, conversational react, self-ask, etc.) which are preconfigured prompt templates guiding the LLM’s behavior. You typically don’t have to write the full prompt logic; LangChain has it under the hood. Instead, you configure the agent by selecting a type and providing the tools.
Basic Example: Let’s create a simple math & search agent with LangChain. This agent can answer questions by either doing calculations or searching the web:
from langchain.agents import initialize_agent, Tool
from langchain.llms import OpenAI
from langchain.utilities import SerpAPIWrapper
# Define a search tool using SerpAPI (for web search results)
search = SerpAPIWrapper() # requires SerpAPI key env var
tools = [
Tool(name="Search", func=search.run, description="useful for searching the web"),
]
# Initialize an OpenAI LLM (text-Davinci or GPT-3.5, etc.)
llm = OpenAI(temperature=0, openai_api_key="YOUR_OPENAI_KEY")
# Create an agent with the tools and LLM, using a standard question-answer agent type
agent = initialize_agent(tools, llm, agent="zero-shot-react-description", verbose=True)
# Run the agent on a query
result = agent.run("Who won the FIFA World Cup in 2010 and what is 18 divided by 6?")
print(result)
In this code, initialize_agent
sets up a Zero-Shot ReAct agent, which means the LLM will decide on the fly which tool to use. We gave it one tool: “Search”. If the question requires math, interestingly this agent doesn’t have a direct calculator tool, but the LLM might use the Search tool to find the math result or solve it by itself. (LangChain also has a built-in calculator tool we could include.) The verbose=True
flag will print out the agent’s thought process. When you run this, you’d see something like:
Thought: The question has two parts: who won 2010 World Cup, and a division.
Action: Search for "2010 World Cup winner"
Observation: "Spain won the 2010 World Cup..."
Thought: 18 divided by 6 is straightforward math = 3.
Action: (no tool needed, directly answer)
Final Answer: "Spain won the 2010 World Cup, and 18 divided by 6 is 3."
LangChain took care of formatting the prompt for the LLM and parsing its output into actions.
When to use LangChain: LangChain is a great general-purpose choice, especially if you need lots of integrations and want quick results using well-tested agent types. It has a large community and many examples available. If you’re building an MVP of an agent, LangChain’s high-level API can get you there fastest. However, LangChain’s abstraction might feel “black-boxed” at times (though you can dig into prompt templates if needed), and it may be less flexible for very custom agent logic compared to lower-level frameworks. Performance-wise, LangChain added support for async and streaming, but some very complex or long-running workflows might require augmentation with something like LangGraph.
LangGraph
What it is: LangGraph is an extension to LangChain aimed at building more controllable and stateful agents. Think of LangGraph as giving you the building blocks to design custom agent orchestration flows (graphs) rather than using only monolithic agent executors. It was developed to address scenarios where reliability and customization are paramount – for example, long-term memory, specific multi-agent routing, or human-in-the-loop checkpoints. LangGraph allows you to define agents and their interactions as a directed graph of nodes, enabling parallelism and complex control flows.
Setup: Install via pip:
pip install langgraph
LangGraph requires Python 3.10+ and you should have LangChain as well (it may get installed as a dependency). If you plan to use it with certain LLM providers (like Anthropic or others), make sure to install those extras or packages (pip install langchain[anthropic]
etc.) as needed.
Core Concepts: The key idea in LangGraph is the Agent = Node in a graph. You can wire nodes such that the output or state of one agent flows to the input of another. It supports patterns like the ones discussed in multi-agent architectures: e.g., a network or a supervisor architecture can be constructed by connecting nodes accordingly. LangGraph also provides some prebuilt agents (similar to LangChain’s agent types) for convenience, like a ReAct agent node you can drop in. Another concept is GraphMemory – a way to persist and share memory across the graph’s execution. Because LangGraph is lower-level, you have to be explicit about the orchestration, but you gain fine control. It’s used by companies like Replit, Uber, LinkedIn for production AI agents, which speaks to its capability for complex scenarios.
Basic Example: Let’s do a quick example with LangGraph by creating a simple ReAct agent node. (This example is from the official docs where they create a weather lookup agent.)
from llama_index.agent import FunctionAgent
from llama_index.tools import Tool
# Define tools as simple Python functions
def multiply(a: float, b: float) -> float:
"""Multiply two numbers."""
return a * b
def add(a: float, b: float) -> float:
"""Add two numbers."""
return a + b
# Wrap functions in Tool objects (LlamaIndex might also accept raw functions)
tools = [
Tool.from_function(multiply),
Tool.from_function(add)
]
# Initialize an LLM (using OpenAI gpt-3.5 turbo model here)
from llama_index import LLMPredictor, ServiceContext
from langchain.chat_models import ChatOpenAI
llm_predictor = LLMPredictor(llm=ChatOpenAI(model="gpt-3.5-turbo", temperature=0))
service_context = ServiceContext.from_defaults(llm_predictor=llm_predictor)
# Create the agent with the tools
agent = FunctionAgent.from_tools(
tools=tools,
llm=llm_predictor,
system_prompt="You are a helpful math agent that can add or multiply numbers using provided tools."
)
# Ask the agent a question
query = "What is 5 + 7, then multiplied by 2?"
response = agent.run(query)
print(response)
In this code, FunctionAgent
is a type of agent that expects to use Python function tools (hence the name). We provided two tools: add
and multiply
. The system prompt guides the agent to use those tools for math. When we call agent.run(query)
, the agent will parse the question “5 + 7, then that multiplied by 2”. Likely, it will first use the add tool on 5 and 7 (getting 12), then use the multiply tool on 12 and 2 (getting 24), and return 24
as the final answer. Under the hood, LlamaIndex’s agent sees that it has tool functions with certain signatures, and it uses the function calling ability of the LLM (OpenAI’s functions or a similar mechanism) to invoke them stepwise.
This example is extremely simple (just to show usage of FunctionAgent
). In real scenarios, you might have tools like query_index
or search_api
. For instance, if you had loaded a corpus of text into LlamaIndex, you could have a tool that does a semantic search in that corpus to retrieve relevant info. The agent could then combine that info to answer a question – that’s an Agentic RAG (Retrieval Augmented Generation) use case, which LlamaIndex excels at. The docs mention building an agentic research assistant over your data as a primary use case.
Choosing LlamaIndex: You’d pick LlamaIndex when your agent needs to be deeply integrated with custom data or knowledge bases. It’s the “go-to” framework for building things like a company internal chatbot that can not only chat but also perform actions like look up data, generate reports with data, etc. It’s also very handy if you want to leverage a lot of the indexing and retrieval primitives (it can connect to SQL, Pandas dataframes, Notion databases, and more as tools). If your use case is “AI agent that knows about my data and can act on it,” LlamaIndex provides a unified solution: data ingestion + agent reasoning.
Compared to LangChain, LlamaIndex might feel more specialized – it’s especially powerful for query-oriented tasks and multi-step workflows involving data. It also has an event-driven workflow system if you need to customize the chain of events when an agent runs. LlamaIndex is under active development, so ensure you reference the latest docs (the agent API has evolved). But overall, it’s a strong choice for knowledge assistants and complex decision-making agents that rely on external information.
CrewAI
What it is: CrewAI is a framework designed for multi-agent orchestration and collaborative agents from the ground up. If LangChain is one-agent-centric, CrewAI is many-agent-centric. The name hints at a “crew” of AI agents working together. CrewAI aims to provide both high-level simplicity and low-level control, with a strong emphasis on concurrency and event-driven workflows. It’s independent of LangChain (built from scratch) and optimized for performance (advertised as “lightning-fast”). CrewAI introduces concepts like Crews (groups of agents) and Flows (orchestration logic) to make managing multiple agents easier.
Setup: Install via pip:
pip install crewai
CrewAI might require Python 3.10+ as well. After installing, you can import and start defining agents, tasks, and flows.
Core Concepts: The two primary abstractions in CrewAI are Agent and Task/Crew:
- An Agent in CrewAI is a role-playing autonomous entity, often defined by a set of abilities (tools) and objectives. Each agent can have its own LLM or share an LLM, and a profile that defines what it’s responsible for.
- A Crew is a collection of agents that work together (collaborative intelligence). You can think of a Crew as the multi-agent system itself. Within a Crew, agents communicate through messages or tasks.
- Flows in CrewAI are about orchestration: you can define a sequence or conditional flow of tasks between agents. CrewAI supports event-driven designs, meaning an agent can produce events that trigger other agents or actions (this is useful for complex workflows where order isn’t just linear).
- CrewAI also emphasizes single LLM calls for multiple steps – meaning it tries to optimize how often the LLM is invoked. In some configurations, it can bundle decisions to reduce API calls (improving speed and cost).
- CrewAI Enterprise (the hosted platform) adds features like monitoring, but as developers we focus on the open-source library.
Basic Example: Let’s illustrate a simple CrewAI usage. Suppose we want two agents: one agent will generate an idea for a social media post, and another agent will critique it. They will then together produce a refined post. This is a mini collaboration scenario:
from crewai import Agent, Crew, Task
# Define our two agents with simple behaviors via system prompts
creative_agent = Agent(
name="Creator",
system_message="You are a creative copywriter agent. Come up with a social media post idea on a given topic.",
)
critic_agent = Agent(
name="Critic",
system_message="You are a critical analyst agent. You will receive a draft post and provide suggestions to improve it.",
)
# Now define how the Crew (interaction) works
crew = Crew(agents=[creative_agent, critic_agent])
# We'll create a simple flow: Creator -> Critic -> Creator
@crew.flow(start="Creator")
def creative_process(message, crew: Crew):
if crew.current_agent.name == "Creator":
# Creator agent got the initial user message as context and produces an idea
idea = crew.current_agent.reply(message)
print("Creator's idea:", idea)
# Now send this idea to Critic agent
crew.send(idea, to="Critic")
elif crew.current_agent.name == "Critic":
# Critic agent receives the idea and gives feedback
feedback = crew.current_agent.reply(message)
print("Critic's feedback:", feedback)
# Send feedback back to Creator for refinement
crew.send(feedback, to="Creator", finalize=True) # finalize indicates after Creator responds, we'll stop
# Run the crew process with an initial user prompt topic
crew.run("We need to post about the importance of data security.")
In this pseudocode (CrewAI’s API might differ slightly in reality, but conceptually), we:
- Create two
Agent
instances with distinct roles. - Initialize a
Crew
with these agents. - Define a flow function decorated by
crew.flow(start="Creator")
meaning the process starts with the Creator agent. Inside the function, we check which agent is active (crew.current_agent
).- When Creator is active, we call
reply(message)
to get its output (the idea).- Then we use
crew.send()
to forward that idea to the Critic. - When Critic is active, we do similarly: get feedback, then send it back to Creator. We mark the last send as
finalize=True
to indicate the flow should end after Creator responds
- Then we use
- When Creator is active, we call
The output might look like:
Creator's idea: "Post: '🔒 Data Security is the New Coffee – You Can't Start Your Day Without It! ... (some creative text)'"
Critic's feedback: "That's a fun angle! Maybe add a tip about how to actually stay secure, so readers have a takeaway."
Creator's idea: "Revised Post: '🔒 Data Security is the New Coffee – ... Plus, remember to use strong passwords and two-factor authentication every day!'"
This demonstrates agents taking turns, each leveraging the prompt we gave as their persona to produce or critique content. CrewAI handled routing the messages between them according to the flow logic we defined.
Why/When to use CrewAI: If you aim to build a system with multiple agents collaborating, CrewAI is purpose-built for that. It’s also a good choice if you want more direct control over conversation turns and event handling than what LangChain offers (LangChain has some multi-agent experimental features, but CrewAI is dedicated to it). CrewAI can be used for scenarios like:
- An AI “assembly line” where different agents handle different stages of a process (as we did with create -> critique -> refine).
- Agents with different expertise working together on a user query (like a financial expert agent + legal expert agent both contribute).
- Orchestration of tasks where one agent’s output triggers another agent automatically.
CrewAI’s performance optimizations can be beneficial if you’re running many agents concurrently. And since it’s independent of LangChain, it might integrate better in some enterprise contexts that require a fresh implementation. The learning curve might be a bit higher because you need to think in terms of asynchronous flows and events, but the framework is designed to simplify common patterns (the creators also offer community courses and examples, indicating it’s developer-friendly).
In short, choose CrewAI when a single-agent loop is not enough – when you literally need a crew of AI workers to handle complex, distributed tasks, and you want a robust way to manage that interaction.
SmolAgent (Hugging Face SmolAgents)
What it is: SmolAgents is a lightweight library by Hugging Face that introduces a novel approach to building agents: rather than heavy abstractions or complex planning loops, it leans into the idea of agents that write and execute code (hence “smol” as in minimal) to solve tasks. The philosophy of SmolAgent is minimalism – keep the framework overhead small and let the LLM do the heavy lifting in a transparent way. One of its key distinctions is using “Code-as-Action”: the agent’s actions are literally in the form of Python code, which is executed to use tools or perform calculations. This is different from many frameworks where the agent’s action is an abstract JSON or text instruction that the framework interprets; with SmolAgents, the agent may respond with a snippet of code that calls an API or parses something, and that code is run directly.
Setup: Install via pip:
pip install smolagents
You may also want to have transformers
or use Hugging Face Hub if you plan to use their models. SmolAgents is LLM-agnostic: it can plug into Hugging Face models, OpenAI, etc. It even provides a CLI for quick experiments (you can run smolagent "your query" --model ...
from terminal).
Core Concepts: The main class is often a CodeAgent which uses tools you define as Python functions. Hugging Face described it as “a very simple library that unlocks agentic capabilities for language models”. The design choices are:
- Minimal abstraction: an agent is basically just an LLM and a Python REPL environment to run code.
- Tools in SmolAgents are Python functions decorated with
@tool
. The LLM is prompted in a way that it can output a code calling those functions. - Because the agent writes code, you can actually see exactly what it’s trying to do (making it more debuggable).
- It integrates with Hugging Face Hub and
transformers
, meaning you can easily switch out models or even use local models. They give utility classes likeHfApiModel
(to use HF Inference API or models on Hub) andTransformersModel
(to run local models).
Basic Example: Let’s say we want an agent that can fetch a webpage and count how many times a word appears on that page. We’ll use SmolAgents to achieve this:
from smolagents import tool, CodeAgent, OpenAIServerModel
import requests
# Define a tool to fetch HTML of a URL
@tool
def fetch_url(url: str) -> str:
"""Fetch the HTML content of the given URL and return it as text."""
resp = requests.get(url, timeout=10)
resp.raise_for_status()
return resp.text
# Define a tool to count occurrences of a word in text
@tool
def count_word(text: str, word: str) -> int:
"""Count how many times `word` appears in `text`."""
return text.lower().count(word.lower())
# Initialize an LLM model to power the agent (using OpenAI GPT-4 via API as an example)
model = OpenAIServerModel(model_id="gpt-4", api_base="https://api.openai.com/v1", api_key="OPENAI_API_KEY")
agent = CodeAgent(tools=[fetch_url, count_word], model=model)
# Run the agent on a task:
query = "Find out how many times the word 'data' appears on the Wikipedia page for Artificial Intelligence."
result = agent.run(query)
print(result)
Let’s walk through what happens when agent.run(query)
is called. SmolAgent will prompt GPT-4 with something like: “You have these tools: fetch_url and count_word. You can write Python code to use them. The user’s question: ...”. The LLM might then output:
# The agent might produce code like this as a plan:
html = fetch_url("https://en.wikipedia.org/wiki/Artificial_Intelligence")
count = count_word(html, "data")
print(f"The word 'data' appears {count} times.")
SmolAgent will execute this code in a sandbox. The code calls our fetch_url
(which returns HTML string), then calls count_word
on that HTML and “data”, then prints the answer. The framework captures the print output and returns it as the agent’s result, perhaps with some formatting. So the final printed result might be: “The word 'data' appears 42 times.” (just as an example).
The beauty here: the agent’s decision-making was entirely transparent as Python code. If it made a mistake (say it didn’t handle errors or needed to parse something differently), we could see it directly and adjust. SmolAgents encourages this kind of clarity. Also, because the agent uses actual code, it can leverage any Python libraries in those tools (requests, BeautifulSoup for parsing, etc.), which is very powerful.
Why/When to use SmolAgents: If you’re a developer who prefers understanding the internals and minimizing “magic,” SmolAgents is appealing. It’s also great when your problem naturally involves a lot of procedural logic or computation – the agent can essentially become a coder that writes a short script to solve the problem. As the DataCamp tutorial noted, SmolAgents keeps abstractions minimal and opts for code-based actions over complicated JSON schemas. This can reduce the mental overhead of learning a big framework.
Some scenarios for SmolAgents:
- You have a set of Python functions (APIs, data utils) and you want the LLM to orchestrate them. SmolAgents makes this straightforward.
- You want to leverage local models from Hugging Face and have flexibility in switching between them or calling multiple providers (SmolAgents provides model wrappers to do that easily).
- Rapid prototyping: writing a new tool is just writing a function with a decorator. The agent can then use it. This ease of extension is a plus.
- Education or debugging: because you can see the generated code, it’s easier to trust and verify what the agent does step by step.
One thing to be cautious: executing code from an LLM raises security considerations (you wouldn’t run this with untrusted user input without sandboxing!). But SmolAgents is intended for controlled environments or non-malicious tasks.
Choosing the Right Framework: To wrap up, here’s a quick when-to-choose-what:
- LangChain: Best for general use, quick setup, and if you need lots of integrations (databases, APIs, etc.) without heavy custom work. Large community and examples – good for beginners and standard use cases.
- LangGraph: Choose this if you hit limitations in LangChain’s agents or need complex, reliable multi-step flows. It’s a bit more advanced, but great for building production-grade agents that need custom control (e.g., enterprise workflows with checks).
- LlamaIndex: Ideal when your agent needs to work heavily with your data (documents, knowledge bases). It shines in building knowledgeable agents and offers powerful indexing and retrieval options out of the box.
- CrewAI: Go for CrewAI when designing systems with multiple agents collaborating is a core requirement. If you envision an agent team with different roles tackling a task concurrently or sequentially, CrewAI provides a framework tailored for that scenario.
- SmolAgents: Perfect for those who want a minimalist, code-first approach. If you like the idea of the agent writing code to solve problems or want to quickly integrate a set of Python tools, this is a fun and effective choice. It’s also good when you want to avoid dependency on any one LLM provider – SmolAgents makes it easy to switch between local and API models.
All these frameworks are evolving. They’re not mutually exclusive either – for instance, you might use LangChain for one part of your project and SmolAgents for another micro-agent that does a specific job. The key is understanding your project’s needs: complexity of workflow, type of data, number of agents, and your comfort level with abstraction. With this understanding and the guidance above, you’re ready to pick a framework and start building your first agentic AI application.
Cohorte Team
March 24, 2025