A Step-by-Step Guide to Using the OpenAI Agents SDK
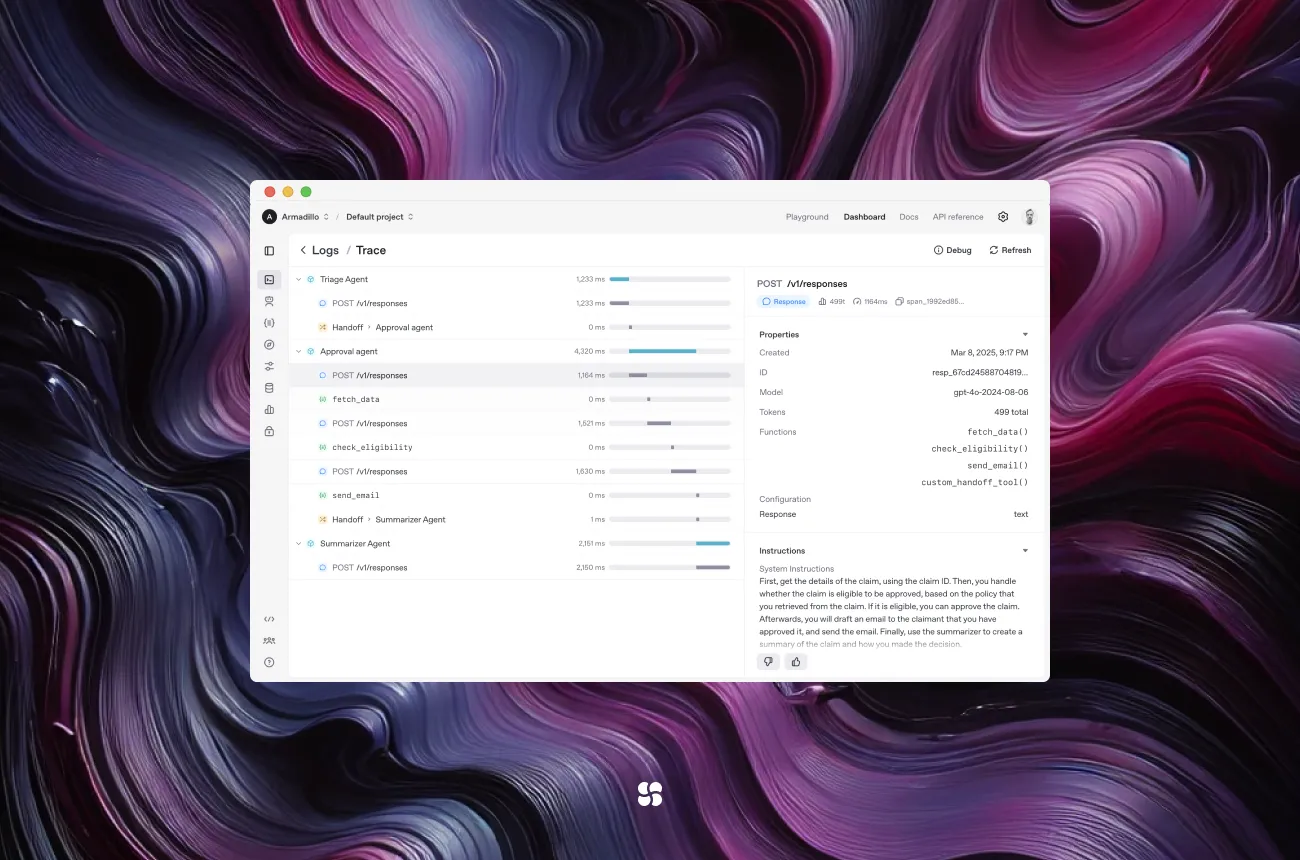
The OpenAI Agents SDK is a powerful framework designed to help developers build sophisticated agentic applications. These applications leverage large language models (LLMs) not just for conversation but to orchestrate actions—whether that’s performing computations, interfacing with external tools, or executing multi-step workflows. This guide presents the framework, its benefits, and walks you through getting started with a practical, code-driven example.
Presentation of the Framework
The Agents SDK provides a modular environment where developers can build agents that “think” and act. At its core, the framework abstracts the complex interactions between multiple AI components. Here are some key features:
• Modular Design: Easily integrate various tools into a unified agent.
• Orchestration: Manage the workflow of multiple agents working together.
• Guardrails & Safety: Built-in mechanisms to enforce safe and predictable agent behavior.
• Extensibility: Adapt the framework to a broad range of applications—from customer support bots to automated research assistants.
This design simplifies the development process, allowing you to focus on the business logic rather than the plumbing of inter-agent communications.
Benefits
Leveraging the OpenAI Agents SDK offers numerous advantages:
• Rapid Prototyping: Quickly build and test agents without extensive boilerplate.
• Enhanced Productivity: Concentrate on the unique functionalities of your application.
• Improved Safety: Enforced guardrails ensure that agents act within defined boundaries.
• Seamless Integration: Easily combine multiple agents and tools for complex workflows.
• Real-Time Interactions: Utilize real-time data and dynamic responses, ideal for applications requiring up-to-date information.
These benefits make the SDK ideal for creating versatile, robust AI systems that adapt to various real-world tasks.
Getting Started
Installation and Setup
Begin by installing the Agents SDK. With a simple pip command, you can add the package to your development environment:
pip install openai-agents-sdk
Once installed, you can import the core classes in your Python script:
from openai_agents_sdk import Agent, Tool
Make sure your OpenAI API key is correctly set up in your environment to authenticate your requests.
First Steps and First Run
A basic agent consists of defining the tools that it will use and creating an instance of the agent. Here’s a minimal example:
# Example: Defining a simple echo tool
class EchoTool(Tool):
def run(self, input_text: str) -> str:
return f"Echo: {input_text}"
# Create an agent instance with the EchoTool
agent = Agent(
name="SimpleAgent",
tools=[EchoTool()]
)
if __name__ == "__main__":
user_input = "Hello, Agent!"
response = agent.run(user_input)
print(response) # Expected output: "Echo: Hello, Agent!"
This simple script demonstrates the core concepts: define a tool, create an agent, and run it to process an input.
Step-by-Step Example: Building a Simple Agent
Let’s build a slightly more advanced agent that can perform two different actions: echoing the input or reversing it. This example shows how you can combine multiple tools within one agent.
1. Define the Tools: Create one tool to echo and another to reverse the input.
2. Instantiate the Agent: Combine the tools into a single agent.
3. Implement a Command Dispatcher: Decide which tool to use based on the input command.
Below is the complete code:
from openai_agents_sdk import Agent, Tool
# Define a simple echo tool
class EchoTool(Tool):
def run(self, input_text: str) -> str:
return f"Echo: {input_text}"
# Define a tool that reverses the input text
class ReverseTool(Tool):
def run(self, input_text: str) -> str:
return input_text[::-1]
# Create an agent that uses both tools
agent = Agent(
name="TransformAgent",
tools=[EchoTool(), ReverseTool()]
)
# Function to choose which tool to use based on the command prefix
def process_input(agent, input_text: str):
if input_text.lower().startswith("echo"):
# Use the EchoTool (assumed to be the first tool)
return agent.tools[0].run(input_text[5:].strip()) # Strip the command part
elif input_text.lower().startswith("reverse"):
# Use the ReverseTool (assumed to be the second tool)
return agent.tools[1].run(input_text[8:].strip()) # Strip the command part
else:
return "Command not recognized. Try 'echo' or 'reverse'."
if __name__ == "__main__":
# Sample inputs for our agent
commands = [
"echo Hello, world!",
"reverse OpenAI",
"unknown test"
]
for cmd in commands:
output = process_input(agent, cmd)
print(f"Input: {cmd}\nOutput: {output}\n")
This example showcases the following:
• Multiple Tools: The agent can perform different actions based on input.
• Command Parsing: A simple dispatcher decides which tool to invoke.
• Modular Design: Easily extend or modify the agent’s behavior by adding new tools.
By building and experimenting with such examples, you’ll gain a strong grasp of how to leverage the Agents SDK to create complex, interactive applications.
Real-World Example: Intelligent Customer Support Agent
In this example, we demonstrate how to build an intelligent customer support agent that handles common customer queries by searching a FAQ database and escalating more complex issues to human support. This real-world use case leverages the Agents SDK to orchestrate multiple tools for a seamless support experience.
Components
• FAQSearchTool: Scans a pre-defined FAQ dataset for relevant answers.
• TicketEscalationTool: Escalates queries to a human support agent when no FAQ match is found.
Implementation
from openai_agents_sdk import Agent, Tool
# Tool to search FAQs
class FAQSearchTool(Tool):
def __init__(self, faq_data):
self.faq_data = faq_data
def run(self, query: str) -> str:
# Simple keyword search in FAQ data
for question, answer in self.faq_data.items():
if query.lower() in question.lower():
return f"FAQ Answer: {answer}"
return "No matching FAQ found."
# Tool to escalate support ticket
class TicketEscalationTool(Tool):
def run(self, query: str) -> str:
# In a real-world scenario, this would create a support ticket.
return "Your query has been escalated to our support team. They will reach out shortly."
# Sample FAQ data
faq_data = {
"How do I reset my password?": "To reset your password, click on 'Forgot Password' on the login page.",
"What is the refund policy?": "Our refund policy lasts 30 days from the purchase date."
}
# Create agent with both tools
support_agent = Agent(
name="SupportAgent",
tools=[FAQSearchTool(faq_data), TicketEscalationTool()]
)
def process_customer_query(agent, query: str) -> str:
# Try to find an FAQ answer first
faq_response = agent.tools[0].run(query)
if "No matching FAQ found" not in faq_response:
return faq_response
else:
# Escalate if no FAQ match is found
return agent.tools[1].run(query)
# Example usage
if __name__ == "__main__":
queries = [
"How do I reset my password?",
"I need help with my account billing."
]
for q in queries:
result = process_customer_query(support_agent, q)
print(f"Query: {q}\nResponse: {result}\n")
Explanation
The FAQSearchTool scans the FAQ dataset to quickly provide an answer for common queries. If no match is found, the query is passed to the TicketEscalationTool, which simulates creating a support ticket for further human intervention. This layered approach ensures that simple issues are resolved instantly while more complex ones are escalated appropriately, showcasing a practical application of the Agents SDK in customer support.
Final Thoughts
The OpenAI Agents SDK is not just about building AI agents—it’s about managing, orchestrating, and scaling them with precision. Its modular design, seamless integration, and built-in safety features let you create powerful systems without the headache. Whether you’re handling customer queries, crunching real-time data, or automating complex workflows, this SDK gives you the building blocks to make it happen.
Time to build.
Cohorte Team
March 14, 2025