A Quick Step-by-Step Guide to Function Calling with Python
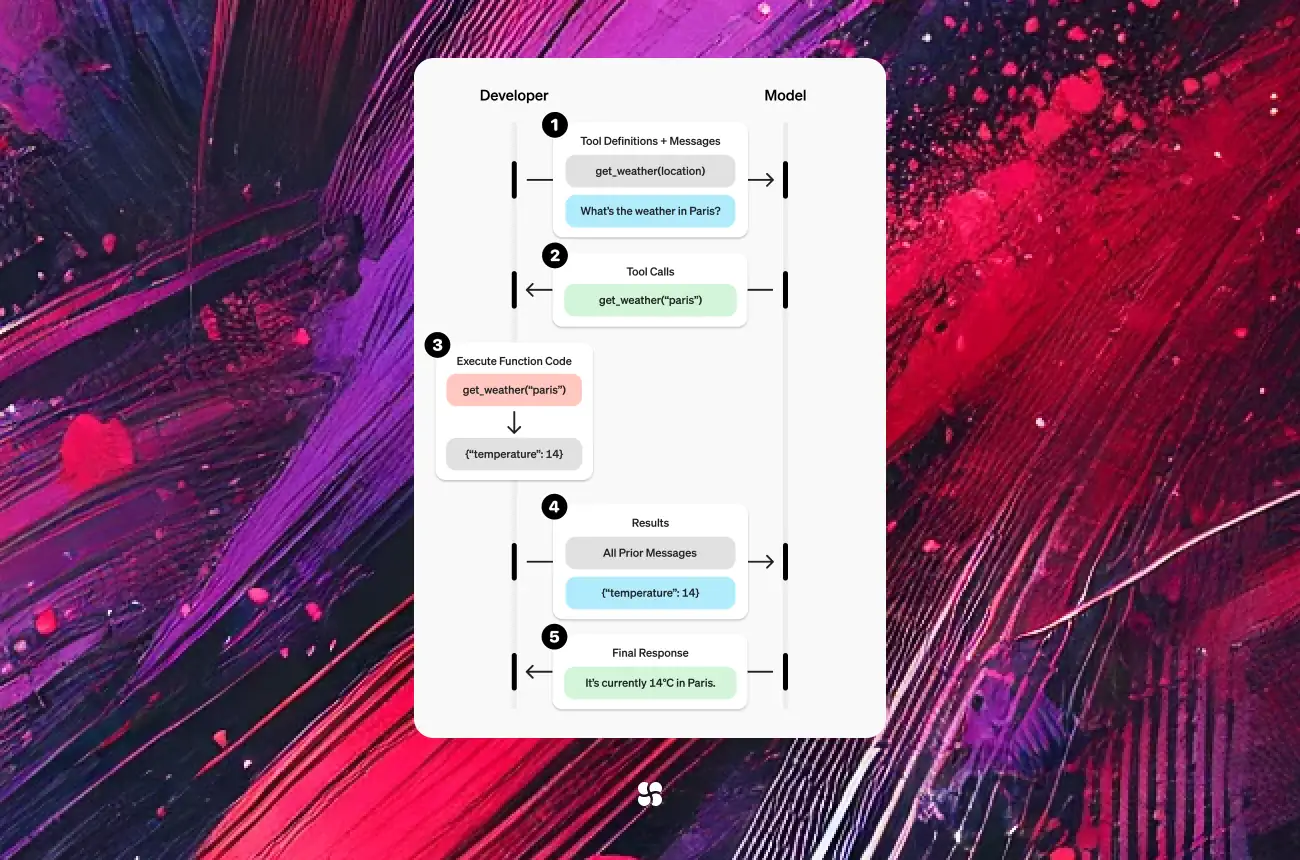
Function calling is a feature that lets you define external functions with a JSON schema. Instead of returning plain text, the LLM can now output structured JSON that maps directly to your custom functions. This makes it much easier to integrate with external APIs, perform computations, or trigger actions based on user input.
Presentation Benefits
Using function calling with GPT‑4o has several key advantages:
- Structured Data Output: By leveraging JSON schemas, you receive data in a consistent and machine-readable format. This eliminates the need for ad hoc parsing of text responses.
- Enhanced Reliability: With explicit function definitions, the model reliably calls the correct function and passes valid parameters.
- Seamless API Integration: Connect your conversational AI directly to external services (weather, finance, messaging, etc.) without extensive prompt engineering.
- Improved Debuggability: With clear function boundaries, developers can quickly identify which part of the conversation led to a particular action, easing troubleshooting.
- Automated Multi-step Workflows: Chain multiple function calls together to create rich, interactive agents that can reason and act autonomously.
Getting Started
1. Installation & Setup
Before diving into the code, ensure you have Python installed and install the OpenAI package:
pip install openai
Next, obtain your OpenAI API key from your OpenAI account and set it up as an environment variable:
import os
os.environ["OPENAI_API_KEY"] = "YOUR_API_KEY"
2. Basic Code Setup
Create a new Python file (e.g., function_calling_guide.py
). The following snippet shows how to initialize the OpenAI client and prepare for function calling:
import openai
import json
# Initialize the OpenAI client using your API key.
openai.api_key = os.environ.get("OPENAI_API_KEY")
# Define a dummy function that our agent can call.
def get_current_weather(location, unit="fahrenheit"):
"""Simulate getting weather data."""
# For demonstration, we return a fixed response.
weather_info = {
"location": location,
"temperature": "72",
"unit": unit,
"forecast": ["sunny", "windy"]
}
return json.dumps(weather_info)
# Define the function specification for the API.
functions = [
{
"name": "get_current_weather",
"description": "Get the current weather in a given location",
"parameters": {
"type": "object",
"properties": {
"location": {
"type": "string",
"description": "The city and state, e.g. San Francisco, CA"
},
"unit": {
"type": "string",
"enum": ["celsius", "fahrenheit"],
"description": "The temperature unit to use"
}
},
"required": ["location"]
}
}
]
Step-by-Step Example: Building a Simple Agent
Now, let’s create a simple agent that uses function calling to respond to a weather inquiry.
Step 1: Send a User Message
Construct a message asking for the weather. The model will decide whether to call a function based on the input.
messages = [
{"role": "user", "content": "What's the weather like in Boston?"}
]
Step 2: Call the Chat Completions API with Function Definitions
Pass the user message along with the function definitions to the API. Notice the function_call
parameter is set to "auto"
—this lets GPT‑4o choose whether to respond directly or output a function call.
response = openai.ChatCompletion.create(
model="gpt-4o", # or use "gpt-4o-2024-11-20" as your version
messages=messages,
functions=functions,
function_call="auto"
)
Step 3: Process the Function Call Response
Check if the model wants to call a function. If so, extract the function name and its arguments from the API’s response.
message = response["choices"][0]["message"]
if message.get("function_call"):
function_name = message["function_call"]["name"]
arguments = json.loads(message["function_call"]["arguments"])
print(f"Function requested: {function_name}")
print(f"With arguments: {arguments}")
# Call the actual function based on the name.
if function_name == "get_current_weather":
function_response = get_current_weather(**arguments)
print("Function Response:", function_response)
else:
# If no function call was made, print the text response.
print("Response:", message.get("content"))
Step 4: (Optional) Feed Function Results Back to GPT
For multi-turn dialogs, you can return the result of the function call back into the conversation. This allows GPT‑4o to summarize or further act on the data.
follow_up_messages = messages + [
message,
{"role": "function", "name": function_name, "content": function_response}
]
final_response = openai.ChatCompletion.create(
model="gpt-4o",
messages=follow_up_messages
)
print("Final GPT Response:", final_response["choices"][0]["message"]["content"])
This step-by-step process demonstrates how GPT‑4o can dynamically decide to call a function and then process the result.
Final Thoughts
OpenAI’s function calling capability revolutionizes how conversational agents can integrate with external tools. By leveraging structured JSON output, developers can build reliable, multi-step workflows with minimal prompt engineering. This feature enhances the precision of responses, making AI applications more robust and interactive.
As you experiment with function calling:
- Explore different functions: Try integrating with real-world APIs for weather, finance, or messaging.
- Chain multiple function calls: Create complex workflows where one function’s output informs the next step.
- Iterate and improve: Use the initial structured output as a base to refine your prompts and function definitions for even better integration.
Function calling with GPT‑4o is a powerful tool for bridging the gap between natural language and actionable code—opening up endless possibilities for creating smart, automated agents.
Happy coding!
Cohorte Team
April 3, 2025